Show List
Creating Jacoco Test Coverage Reports Using Gradle and JUnit
JUnit is a popular framework for writing tests for Java programs. Jacoco stands for Java Code Coverage and is a library used to generate reports on test coverage.
In this example, we will be creating a sample java program, write unit tests for it and then use Gradle to create test coverage report.
Project Structure
Here is the structure of the Gradle project we will be creating. On the IDE you can start with creating a new Gradle Java project or import the source code from the link provided at the end of this tutorial page.
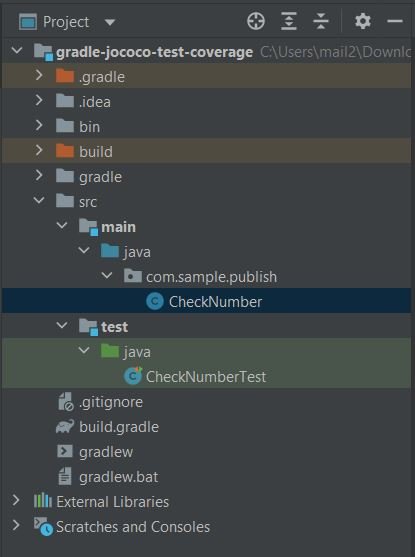
CheckNumber.java
This is our main java class. It has isEven method which returns True or False depending on whether the passed number is even or not.
package com.sample.publish;
public class CheckNumber
{
public boolean isEven(int i) {
if (i % 2 == 0) {
return true;
} else {
return false;
}
}
}
CheckNumberTest.java
This is the test class. It uses JUnit libraries to test the CheckNumber class above for even and odd number values.
import com.sample.publish.CheckNumber;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CheckNumberTest {
CheckNumber cn;
@BeforeEach
void setUp(){
cn = new CheckNumber();
}
@Test
void testEven(){
assertEquals(true, cn.isEven(2) );
}
@Test
void testOdd(){
assertEquals(false, cn.isEven(3) );
}
}
build.gradle
This file lists the plugins, dependencies for the project and repository to download those dependencies. test block configures JUnit to be used for Gradle test task. In the jacocoTestReport we are enabling html report. Jacoco also supports xml and csv report types.
As the Jacoco reports are to be created after tests are run so we have listed jacocoTestReport task to be dependent on test task. finalized by property is used to trigger jacocoTestReport after test task is complete.
apply plugin : "application"
apply plugin: "jacoco"
group 'com.sample'
version '1.0.0'
repositories {
// Define where the dependencies are to be looked for
mavenCentral()
}
dependencies {
//Jars the application is dependent on
testImplementation group: 'junit', name: 'junit', version: '4.13.2'
testImplementation 'org.junit.jupiter:junit-jupiter-engine:5.9.1'
testImplementation 'org.junit.jupiter:junit-jupiter-api:5.9.1'
}
test {
useJUnitPlatform()
}
jacocoTestReport{
reports{
html.enabled true
}
}
jacocoTestReport.dependsOn 'test'
test.finalizedBy jacocoTestReport
Running the "Gradle clean build test" command from the project root will build the application, run the tests and create the Jacoco report.
C:\Users\mail2\Downloads\gradle-jococo-test-coverage>gradle clean build test BUILD SUCCESSFUL in 5s
The generated Jacoco report can be found at location \build\reports\jacoco\test\html
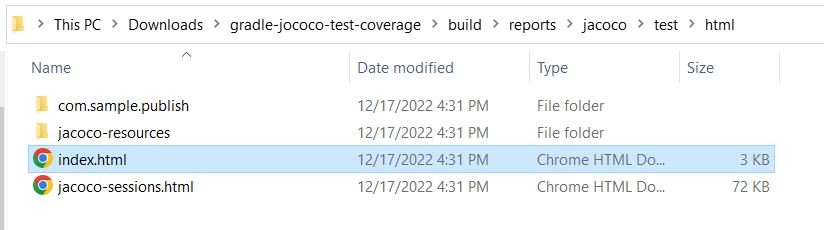
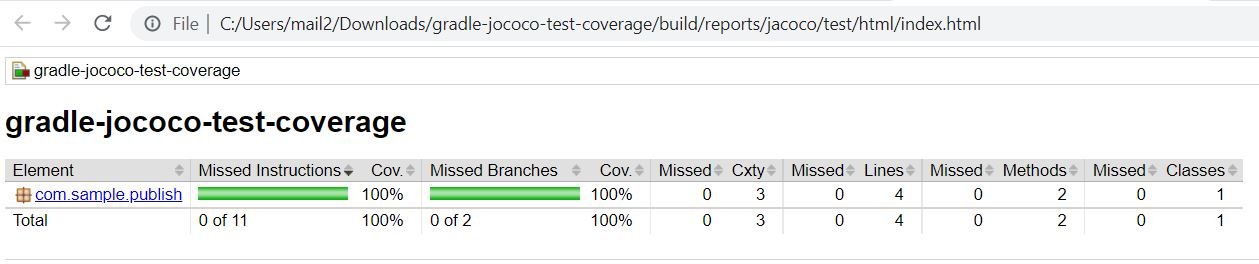
You can click on the links provided on the report to view details.
Source Code:
https://github.com/it-code-lab/gradle-jococo-test-coverage
Leave a Comment