Show List
First Angular Application and Elements Overview
Create a folder for your application in the desired location and open with VS code. Open terminal and run command "ng new first-application"
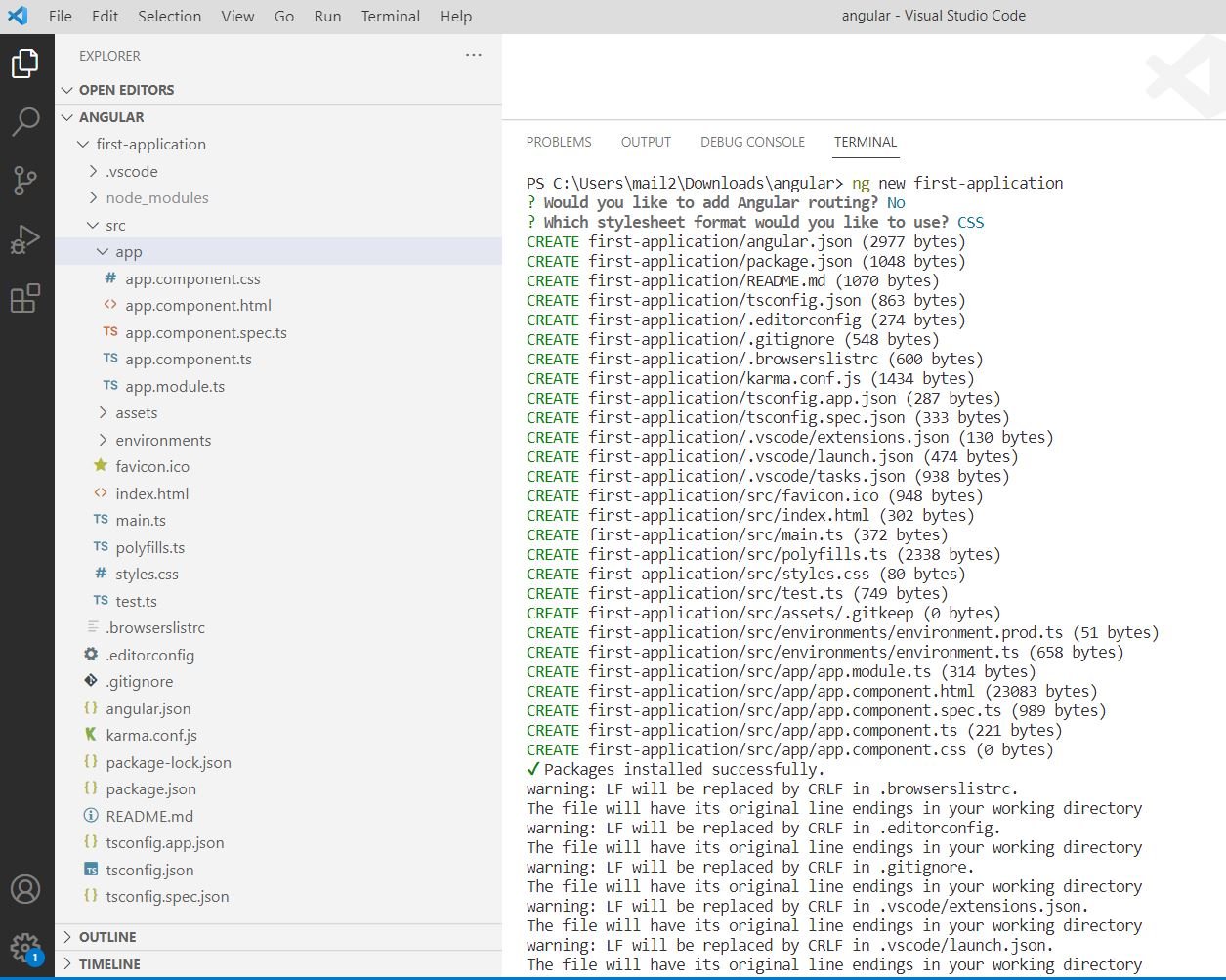
To run the application, change the directory to "first-application" and then run command "ng serve"
PS C:\Users\mail2\Downloads\angular> cd first-application PS C:\Users\mail2\Downloads\angular\first-application> ng serve ✔ Browser application bundle generation complete. Initial Chunk Files | Names | Raw Size vendor.js | vendor | 1.77 MB | polyfills.js | polyfills | 318.07 kB | styles.css, styles.js | styles | 210.10 kB | main.js | main | 48.06 kB | runtime.js | runtime | 6.53 kB | | Initial Total | 2.34 MB Build at: 2022-10-12T14:14:31.558Z - Hash: b767a1ec6f1e5f86 - Time: 27410ms ** Angular Live Development Server is listening on localhost:4200, open your browser on http://localhost:4200/ **
Navigate to url http://localhost:4200/ to view the page
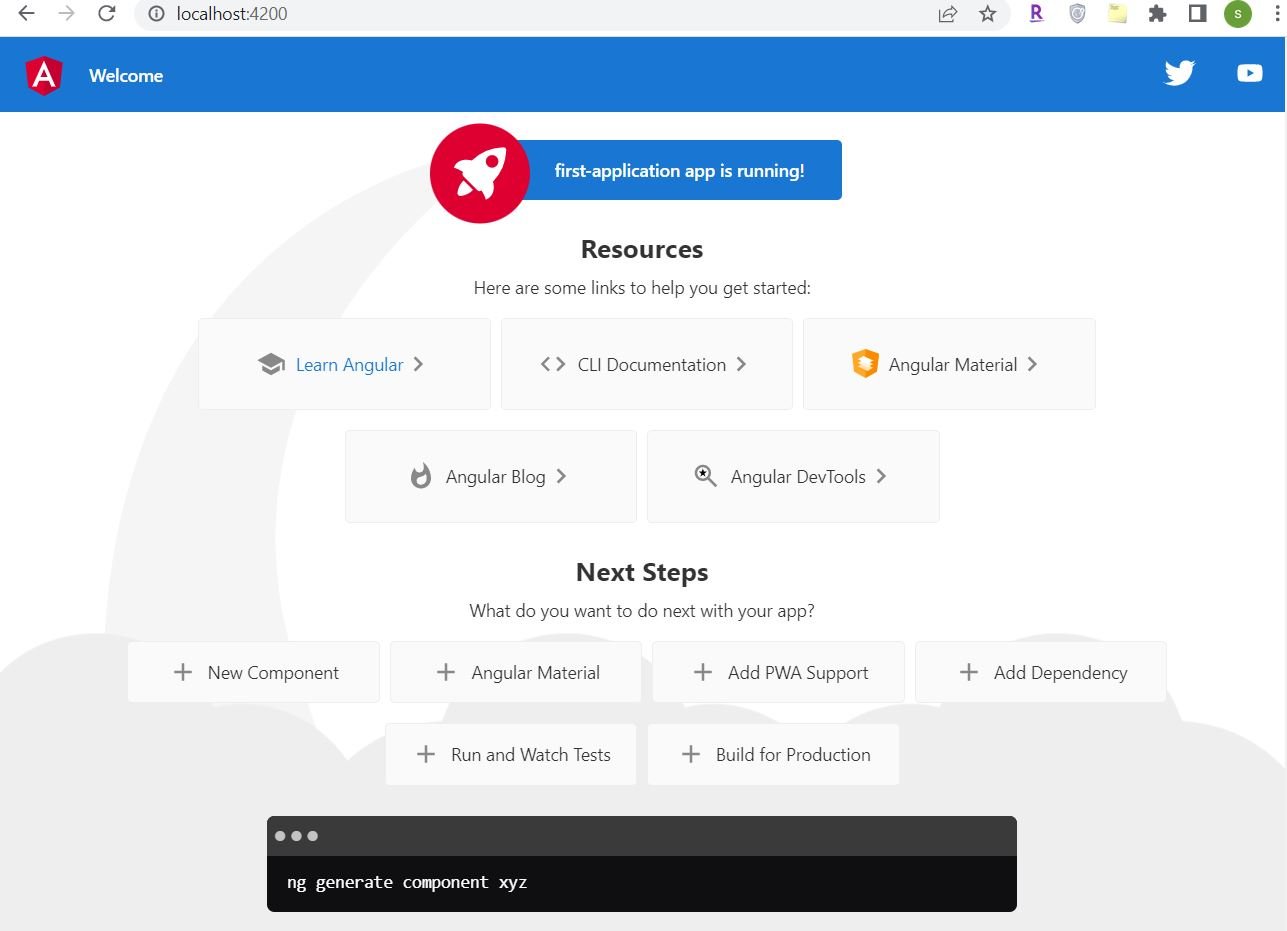
We are going to change the default HTML of the page. Go to the app.component.html file and update the content as below:
app.component.html
<div><h1 id="london">London</h1><p>London, the capital of England and the United Kingdom, is a 21st-century city with history stretching back toRoman times.</p><h1 id="paris">Paris</h1><p>Paris, France's capital, is a major European city and a global center for art, fashion, gastronomy and culture.Its 19th-century cityscape is crisscrossed by wide boulevards and the River Seine.</p><h1 id="tokyo">Tokyo</h1><p>Tokyo, Japan’s busy capital, mixes the ultramodern and the traditional, from neon-lit skyscrapers to historictemples. The opulent Meiji Shinto Shrine is known for its towering gate and surrounding woods.</p></div>
Browser will automatically refresh the content on the url http://localhost:4200/
London
London, the capital of England and the United Kingdom, is a 21st-century city with history stretching back to Roman times.
Paris
Paris, France's capital, is a major European city and a global center for art, fashion, gastronomy and culture. Its 19th-century cityscape is crisscrossed by wide boulevards and the River Seine.
Tokyo
Tokyo, Japan’s busy capital, mixes the ultramodern and the traditional, from neon-lit skyscrapers to historic temples. The opulent Meiji Shinto Shrine is known for its towering gate and surrounding woods.
Angular Application Elements Overview
Here is the overview of key elements of the application.
index.html
This is the landing page for the application. <app-root> is the marker for loading the component. The components and styles are dynamically injected into index.html at run time by ng serve command.
<!doctype html><html lang="en"><head><meta charset="utf-8"><title>FirstApplication</title><base href="/"><meta name="viewport" content="width=device-width, initial-scale=1"><link rel="icon" type="image/x-icon" href="favicon.ico"></head><body><app-root></app-root></body></html>
app.component.ts
Angular components usually have html file, style file and a component.ts file. The component.ts file contains the information about the selector to be used for the component.
import { Component } from '@angular/core';@Component({selector: 'app-root',templateUrl: './app.component.html',styleUrls: ['./app.component.css']})export class AppComponent {title = 'first-application';}
@Component decorator makes a class an Angular component. The decorator has the properties for selector, template and style.
app.module.ts
This file has the core configuration, loads dependencies and declares the components that are used in the application.
import { NgModule } from '@angular/core';import { BrowserModule } from '@angular/platform-browser';import { AppComponent } from './app.component';@NgModule({declarations: [AppComponent],imports: [BrowserModule],providers: [],bootstrap: [AppComponent]})export class AppModule { }
@NgModule decorator makes a class NgModule and supplies configuration data to compile components template and create injectors at runtime.
node_modules
Contains the dependency packages.
package.json
Contains the information about dependencies. These packages get installed in node_modules when npm install command is run
{"name": "first-application","version": "0.0.0","scripts": {"ng": "ng","start": "ng serve","build": "ng build","watch": "ng build --watch --configuration development","test": "ng test"},"private": true,"dependencies": {"@angular/animations": "^14.2.0","@angular/common": "^14.2.0","@angular/compiler": "^14.2.0","@angular/core": "^14.2.0","@angular/forms": "^14.2.0","@angular/platform-browser": "^14.2.0","@angular/platform-browser-dynamic": "^14.2.0","@angular/router": "^14.2.0","rxjs": "~7.5.0","tslib": "^2.3.0","zone.js": "~0.11.4"},"devDependencies": {"@angular-devkit/build-angular": "^14.2.2","@angular/cli": "~14.2.2","@angular/compiler-cli": "^14.2.0","@types/jasmine": "~4.0.0","jasmine-core": "~4.3.0","karma": "~6.4.0","karma-chrome-launcher": "~3.1.0","karma-coverage": "~2.2.0","karma-jasmine": "~5.1.0","karma-jasmine-html-reporter": "~2.0.0","typescript": "~4.7.2"}}
main.ts
It identifies which Angular module is to be loaded when the application starts.
import { enableProdMode } from '@angular/core';import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';import { AppModule } from './app/app.module';import { environment } from './environments/environment';if (environment.production) {enableProdMode();}platformBrowserDynamic().bootstrapModule(AppModule).catch(err => console.error(err));
Source Code:
https://github.com/it-code-lab/angular-application
Leave a Comment