Show List
Event Binding
Invoking a JavaScript function based on the events triggered on the HTML element is called event biding. Events cound be click, dblclick, submit, blur, focus, scroll, cut, copy, paste, keyup, keydown, keypress, mouseup, mousedown, mouseenter, drag, drop etc.
Syntax for event binding is : (event) = "functionToCall(parameter)"
Examples:
- <button (click)="processData()" >Process</button>
- Can pass the parameter if needed. <button (click)="onDisplay(10)">Display</button>
- Can pass the event that can be used to extract information. <button (click)="onShow($event)">Show</button>
- <input (keyup)="onKeyUp($event)">
- <input (keyup.control.shift.enter)="myFunction()">
Binding Button Click Event
In the previous chapter we creates home component and added a button in the HTML. Here we are binding the button click event to the function in TypeScript file so that when the user clicks the button, an alert message gets displayed.
home.component.ts
import { Component, OnInit } from '@angular/core';@Component({selector: 'app-home',templateUrl: './home.component.html',styleUrls: ['./home.component.css']})export class HomeComponent implements OnInit {constructor() { }ngOnInit(): void {}showMsg(){alert("Event Triggered")}}
home.component.html
<button (click)="showMsg()"> Demo </button>
Now on the click of the demo button the alert gets displayed:
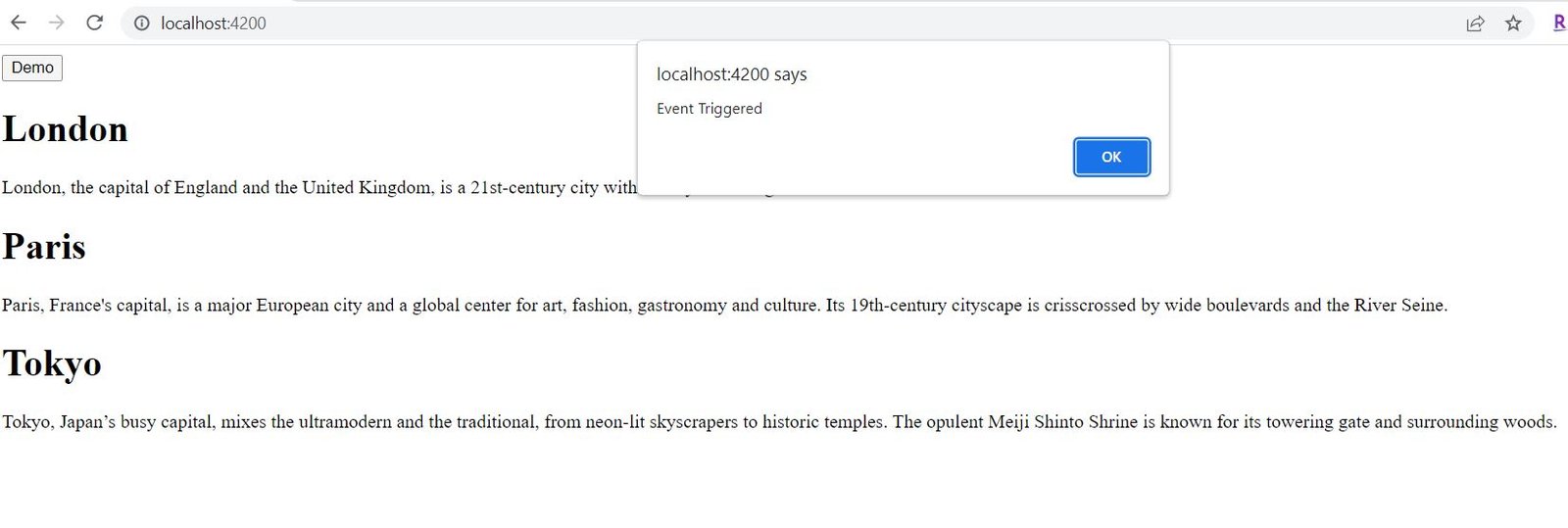
Binding Text Input Event
Lets add a input text element on home component and bind the input event to a function.
home.component.ts
import { Component, OnInit } from '@angular/core';@Component({selector: 'app-home',templateUrl: './home.component.html',styleUrls: ['./home.component.css']})export class HomeComponent implements OnInit {userName: string = "";constructor() { }ngOnInit(): void {this.userName = "";}showMsg(){alert("Event Triggered")}updateUserName(e: any){this.userName = (e.target as HTMLInputElement).value;}}
home.component.html
<button (click)="showMsg()"> Demo </button><br><br><input type="text" (input)="updateUserName($event)"><br>{{userName}}
When the text is entered in the input field, it gets updated under the input element.
London
London, the capital of England and the United Kingdom, is a 21st-century city with history stretching back to Roman times.
Paris
Paris, France's capital, is a major European city and a global center for art, fashion, gastronomy and culture. Its 19th-century cityscape is crisscrossed by wide boulevards and the River Seine.
Tokyo
Tokyo, Japan’s busy capital, mixes the ult
Source Code:
https://github.com/it-code-lab/angular-application
Leave a Comment