Show List
Angular Service
Service is a reusable piece of code that provides specific functionality. Sharing logic or data across multiple components, fetching back end data, logging are some of the scenarios where services are used.
Create Service
Service can be created by using Angular CLI command "ng generate service serviceName" or in short "ng g s serviceName"
PS C:\Users\mail2\Downloads\angular\first-application> ng g s product CREATE src/app/product.service.spec.ts (398 bytes) CREATE src/app/product.service.ts (143 bytes)
product.service.ts
Service is a class with @Injectable decorator. Below is the default code generated for the service by the CLI
import { Injectable } from '@angular/core';@Injectable({providedIn: 'root'})export class ProductServiceService {constructor() { }}
Update the code to as below. This service now has a function to return the product list from a hard coded array.
import { Injectable } from '@angular/core';import { Product } from './product';@Injectable({providedIn: 'root'})export class ProductService {constructor() { }public getProducts: Product[] = [new Product(1, 'ProductA', 10),new Product(2, 'ProductB', 100),new Product(3, 'ProductC', 500),new Product(4, 'ProductC', 900),new Product(5, 'ProductC', 1200),new Product(6, 'ProductC', 1500),new Product(7, 'ProductC', 5000),]}
product.ts
This class is used as a model in above service.
export class Product {constructor(productID: number, name: string, price: number) {this.productID = productID;this.name = name;this.price = price;}productID: number;name: string;price: number;}
Using the Service
home.component.ts
Service is injected through the constructor.
import { Component, OnInit } from '@angular/core';import { Product } from '../product';import { ProductService } from '../product.service';@Component({selector: 'app-home',templateUrl: './home.component.html',styleUrls: ['./home.component.css']})export class HomeComponent implements OnInit {products: any ;maxPrice:any;constructor(private ps:ProductService) { }ngOnInit(): void {}getProducts(){this.products = this.ps.getProducts;}}
home.component.html
Service is called to display the products that are under the price limit provide.
Price Limit: <input type="text" [(ngModel)]="maxPrice"><br><br><button (click)="getProducts()"> Display Products </button><br><br><table class='table'><thead *ngIf="products.length > 0"><tr><th>ID</th><th>Name</th><th>Price</th></tr></thead><tbody><tr *ngFor="let product of products;"><ng-container *ngIf="product.price <= maxPrice"><td>{{product.productID}}</td><td>{{product.name}}</td><td>{{product.price}}</td></ng-container></tr></tbody></table>
Output:
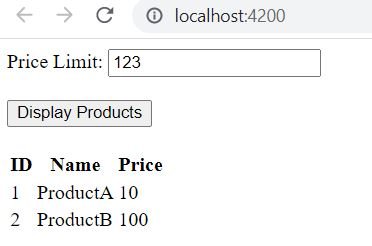
Source code
https://github.com/it-code-lab/angular-application
Leave a Comment