Show List
Rest API using Node js Express and Mongo DB
In this example we will create REST API. Mongo DB will be used as database. Express framework will be used to handle the requests.
Here is the project structure:
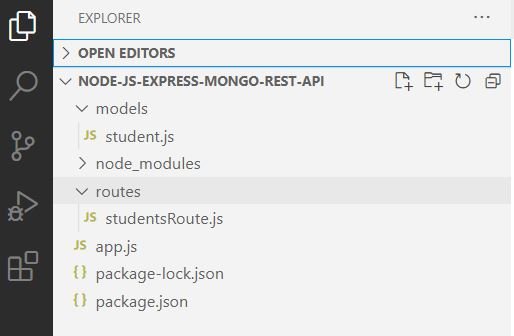
Mongo DB Installation
If you do not have Mongo DB installed on your computer, you can install the community version from official site https://www.mongodb.com/try/download/community2
During the installation steps, select Compass to be installed as well. Compass is GUI to query the Mongo DB data.
After installation is successful, create database with name studentsdb. This database will be used to store students information.
As the DB is running on port 27017 url to connect to the studentsdb database would be:
mongodb://localhost:27017/studentdb
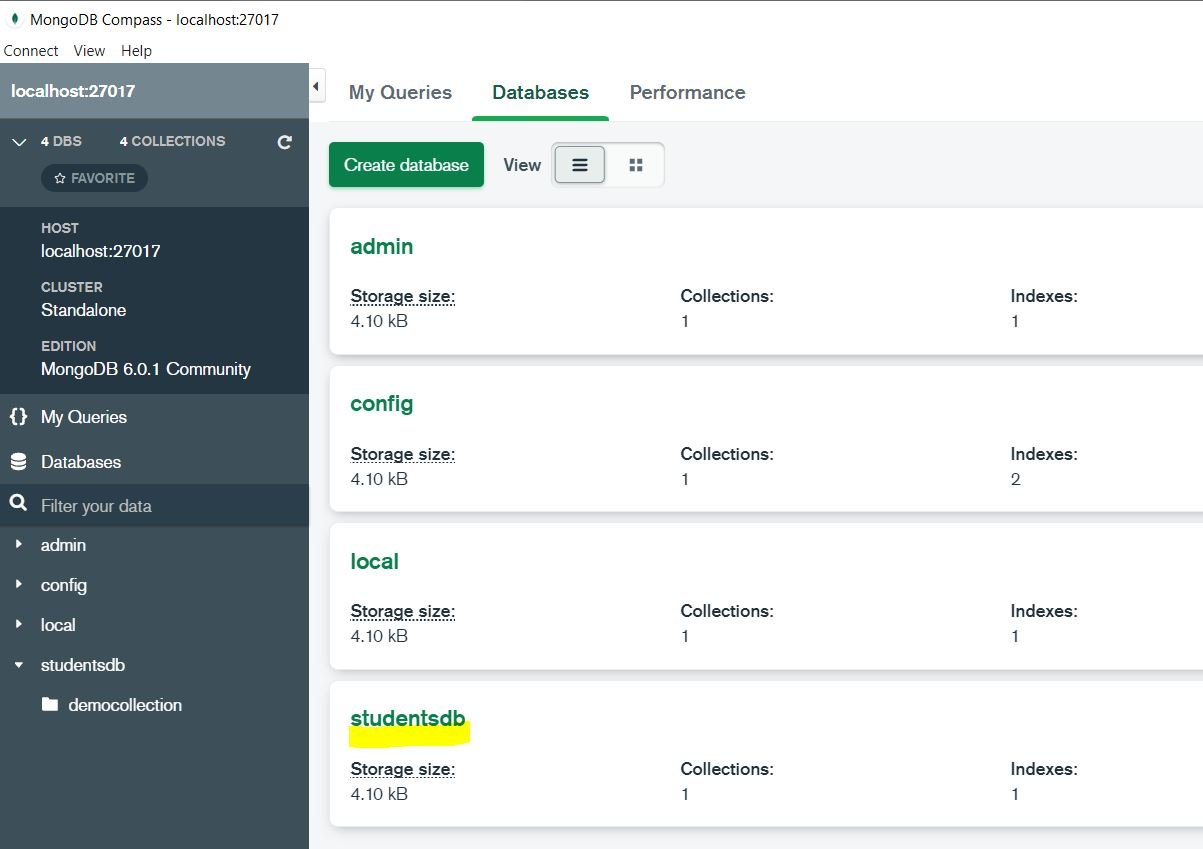
Set Up
Create a new folder with name node-js-express-mongo-rest-api and open in VS Code. Run below commands from the terminal:
- npm init : Provide the project name etc or select default. This command will generate package.json file
- npm i express mongoose: This command will install express (framework to handle http calls) and mongoose (to work with Mongo DB)
- npm install --save-dev nodemon: This command will install nodemon (to automatically restart the application when file changes are detected) as dev dependency. To start the application with nodmon, we will have the start command to include nodeman. So when the npm start is run on the application, app.js file will run with nodemon.
Package.json:
{"name": "js-express-mongo-rest-api","version": "1.0.0","description": "","main": "index.js","scripts": {"start": "nodemon app.js"},"author": "","license": "ISC","dependencies": {"express": "^4.18.1","mongoose": "^6.6.1"},"devDependencies": {"nodemon": "^2.0.20"}}
App.js
This file connects to the database, starts the server and transfers all the requests from students end point to studentsRoute
const express = require('express')
const mongoose = require('mongoose')
const url = 'mongodb://localhost:27017/studentdb'
const app = express()
mongoose.connect(url, {useNewUrlParser:true})
const con = mongoose.connection
con.on('open', () => {
console.log('connected to mongo db...')
})
//Convert request data to Json
app.use(express.json())
const studentRouter = require('./routes/studentsRoute')
// Transfer all the requests from students endpoint to studentRouter
app.use('/students',studentRouter)
//Start the server
app.listen(8080, () => {
console.log('Server started')
})
studentsRoute.js
This route file handles get, delete, post and patch http operations and uses mongoose model object to interact with the database.
const express = require('express')const router = express.Router()const student = require('../models/student')router.get('/', async (req, res) => {try {const students = await student.find()res.json(students)} catch (err) {res.send('Error ' + err)}})router.get('/:id', async (req, res) => {try {const student = await student.findById(req.params.id)res.json(student)} catch (err) {res.send('Error ' + err)}})router.delete('/:id', async (req, res) => {try {const student = await Model.findByIdAndDelete(req.params.id)res.send(`Document with ${student.name} has been deleted..`)} catch (err) {res.send('Error ' + err)}})router.post('/', async (req, res) => {try {const st = new student({name: req.body.name,grade: req.body.grade})const a1 = await st.save()res.json(a1)} catch (err) {console.log(err)res.send('Error')}})router.patch('/:id', async (req, res) => {try {const student = await student.findById(req.params.id)student.name = req.body.namestudent.grade = req.body.gradeconst a1 = await student.save()res.json(a1)} catch (err) {res.send('Error')}})module.exports = router
student.js
This file exports mongoose model. Mongoose model is a wrapper on the DB schema. It provides an interface for creating, querying, updating, deleting records in the database.
const mongoose = require('mongoose')const studentSchema = new mongoose.Schema({name: {type: String,required: true},grade: {type: String,required: true}})module.exports = mongoose.model('student',studentSchema)
Running the code:
PS E:\Desktop\node-js-express-mongo-rest-api> npm start > js-express-mongo-rest-api@1.0.0 start > nodemon app.js [nodemon] 2.0.20 [nodemon] to restart at any time, enter `rs` [nodemon] watching path(s): *.* [nodemon] watching extensions: js,mjs,json [nodemon] starting `node app.js` Server started connected to mongo db...
The API can be accessed from Postman:
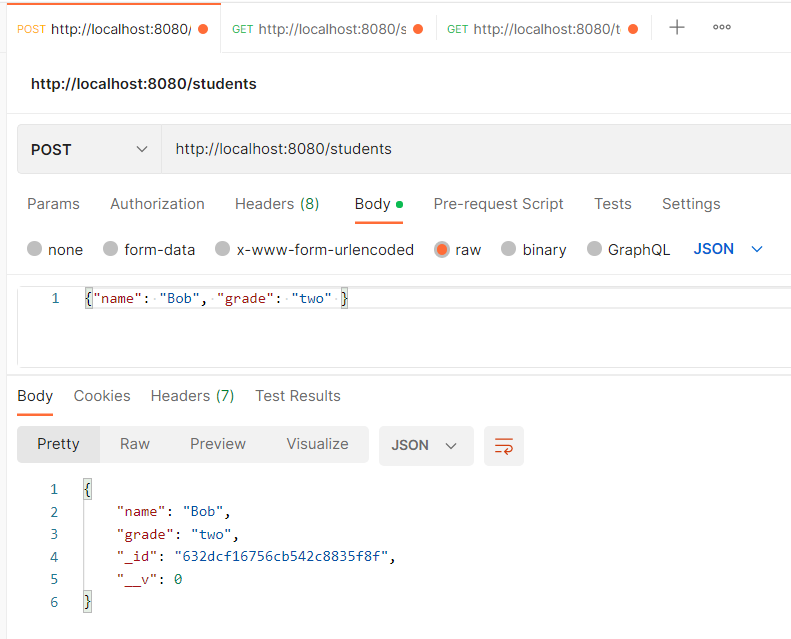
Source Code:
https://github.com/it-code-lab/node-js-express-mongo-rest-api
Leave a Comment