Show List
Springboot RabbitMQ Demo
In this example we will send/receive message to the RabbitMQ using Springboot application.
From the Spring website create a project with RabbitMQ, Spring Web and Lombok dependencies.
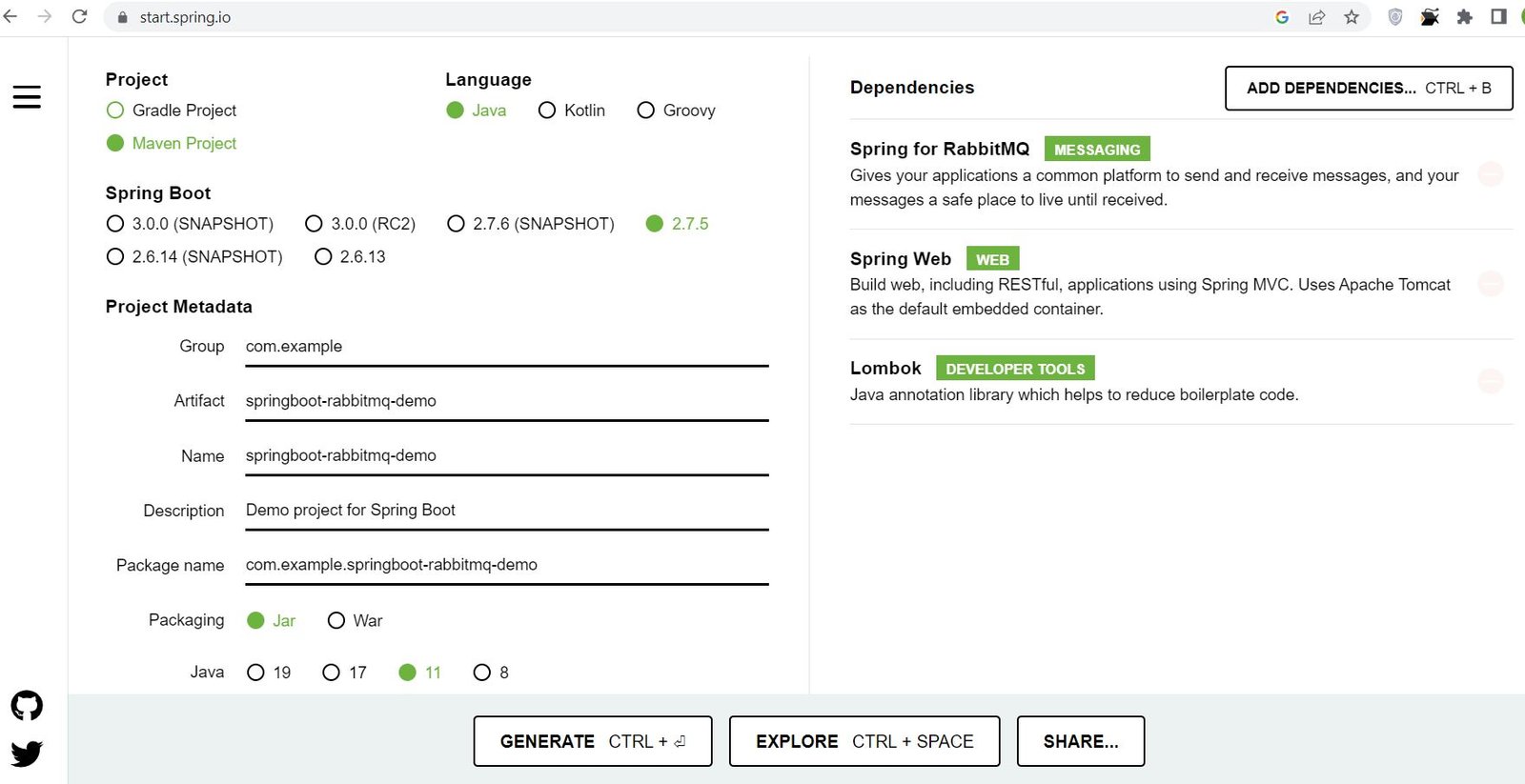
Download project, extract and open with an IDE. I am using intellij.
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.5</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>springboot-rabbitmq-demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-rabbitmq-demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.amqp</groupId>
<artifactId>spring-rabbit-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
Message Publisher
We are going to use a rest controller trigger message to RabbitMQ. This controller has a get method to accept student name and then will build student object to send to the queue. RabbitTemplate object is provided by Springboot.
MQController.java
package com.example.springbootrabbitmqdemo.controller;
import com.example.springbootrabbitmqdemo.model.Student;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MQController {
@Autowired
RabbitTemplate rabbitTemplate;
@GetMapping("/student/{studentName}")
private void getMessage(@PathVariable String studentName){
Student st = new Student(1, studentName);
rabbitTemplate.convertAndSend("Green", st);
rabbitTemplate.convertAndSend("topic-exchange-demo","red.blue", st);
}
}
We are sending one message to the "Green" queue directly. And another message is sent to a topic exchange which routes the messages to "Red" and "Blue" queues.
Student.java
package com.example.springbootrabbitmqdemo.model;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.io.Serializable;
@AllArgsConstructor
@NoArgsConstructor
@Data
public class Student implements Serializable {
private int student_id;
private String name;
}
application.properties
spring.rabbitmq.host=localhost
spring.rabbitmq.port=5672
When the application is run and user sends the request to student endpoint (example below) then messages get sent to the RabbitMQ queues.
http://localhost:8080/student/bob
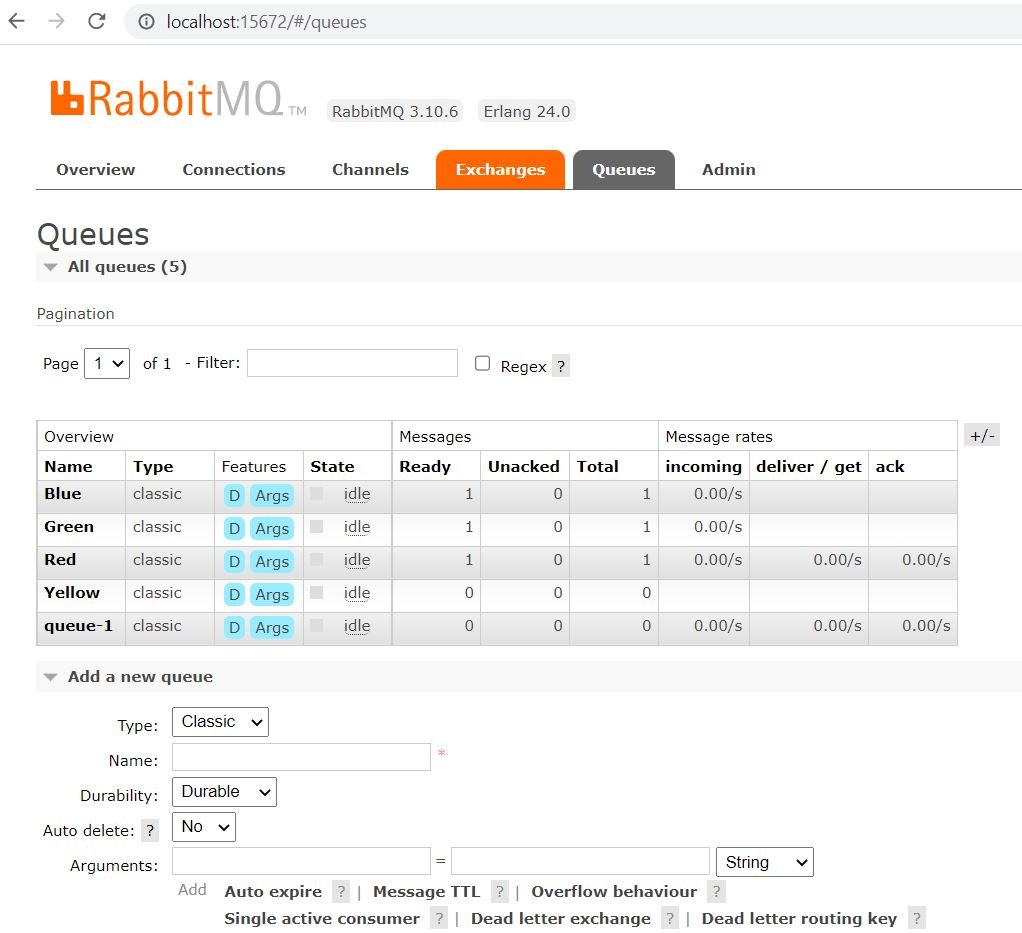
Message Listener
We are creating the message listener as service. @RabbitListner annotation is used to listen to a queue.
MQListener.java
package com.example.springbootrabbitmqdemo.service;
import com.example.springbootrabbitmqdemo.model.Student;
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Service;
@Service
public class MQListener {
@RabbitListener(queues="Red")
public void getMessageRed(Student student){
System.out.println("Received message in Red queue for student - " + student.getName() );
}
@RabbitListener(queues="Blue")
public void getMessageBlue(Student student){
System.out.println("Received message in Blue queue for student - " + student.getName() );
}
@RabbitListener(queues="Green")
public void getMessageGreen(Student student){
System.out.println("Received message in Green queue for student - " + student.getName() );
}
}
Now rerunning the application would create the instance of listener service and the messages would get consumed.
2022-11-12 15:08:53.486 INFO 339056 --- [ main] c.e.s.SpringbootRabbitmqDemoApplication : Started SpringbootRabbitmqDemoApplication in 3.566 seconds (JVM running for 4.176) Received message in Green queue for student - bob Received message in Blue queue for student - bob Received message in Red queue for student - bob
Source code:
https://github.com/it-code-lab/springboot-rabbitmq-demo
Leave a Comment