Show List
Sample Ant Build File
As we discussed earlier Ant is used to automate the build and deployment steps. Here is an example where we will use Ant script to compile java classes, create jar file and then run the jar.
Ant uses xml script to identify the tasks to be executed. So we will create that script and we will use two Java classes to build the jar.
Java Classes
HelloWorld.java
public class HelloWorld{public static void main(String args[]){System.out.println("Hello World");}}
Test.java
public class Test {public static void main(String args[]) {System.out.println("Test Class says hello.");}}
build.xml
Here is the sample build.xml file that will be executed by Ant. The default name for the Ant configuration file is build.xml. To run this file we just have to run command "ant" from the location where build.xml file is present. If the file had a different name (for example config.xml), we would run the command "ant -buildfile config.xml"
The Java classes mentioned above are present in the sub directory "src"
Here is a quick breakdown of different parts in the build.xml
- As it is a xml file, starts with xml declaration statement.
- Build.xml should contain only one project element. This contains all other elements. "default" is required attribute for project element to identify the target to be called when script is executed by Ant command. "basedir" is the directory from which all relative paths are resolved.
- Properties are like constants defined for later use in the script.
- Targets are group of tasks and may have "depends" attribute. Targets listed in the "depends" are executed before the dependent target.
- There are some built-in core Ant tasks. In the script below we have delete, mkdir, javac, jar, java, manifest are built-in task examples.
- When the Ant command is run it calls the "run" target. Going through the dependencies, the order of execution of the targets is clean -> compile -> jar -> run
<?xml version="1.0" ?><project name="FirstProject" basedir="." default="run"><!-- Property names going to be used in the tasks below. --><property name="src.dir" value="src"/><property name="build.dir" value="build"/><property name="classes.dir" value="${build.dir}/classes"/><property name="jar.dir" value="${build.dir}/jar"/><property name="main-class" value="HelloWorld"/><target name="clean"><!-- Task to delete directory --><delete dir="${build.dir}"/></target><target name="compile" depends="clean"><!-- Task to create directory --><mkdir dir="${classes.dir}"/><!-- Task to compile java classes --><javac srcdir="${src.dir}" destdir="${classes.dir}"/></target><target name="jar" depends="compile"><!-- Task to create directory --><mkdir dir="${jar.dir}"/><!-- Task to create jar file with Main-Class assignment --><jar destfile="${jar.dir}/${ant.project.name}.jar" basedir="${classes.dir}"><manifest><attribute name="Main-Class" value="${main-class}"/></manifest></jar></target><target name="run" depends="jar"><!-- Task to run the jar. Fork value true enables class execution in another JVM--><java jar="${jar.dir}/${ant.project.name}.jar" fork="true"/></target></project>
Ant execution results:
PS E:\Desktop\del\Ant-2> ant Buildfile: E:\Desktop\del\Ant-2\build.xml clean: compile: [mkdir] Created dir: E:\Desktop\del\Ant-2\build\classes [javac] E:\Desktop\del\Ant-2\build.xml:20: warning: 'includeantruntime' was not set, defaulting to build.sysclasspath=last; set to false for repeatable builds [javac] Compiling 2 source files to E:\Desktop\del\Ant-2\build\classes jar: [mkdir] Created dir: E:\Desktop\del\Ant-2\build\jar [jar] Building jar: E:\Desktop\del\Ant-2\build\jar\FirstProject.jar run: [java] Hello World BUILD SUCCESSFUL Total time: 0 seconds
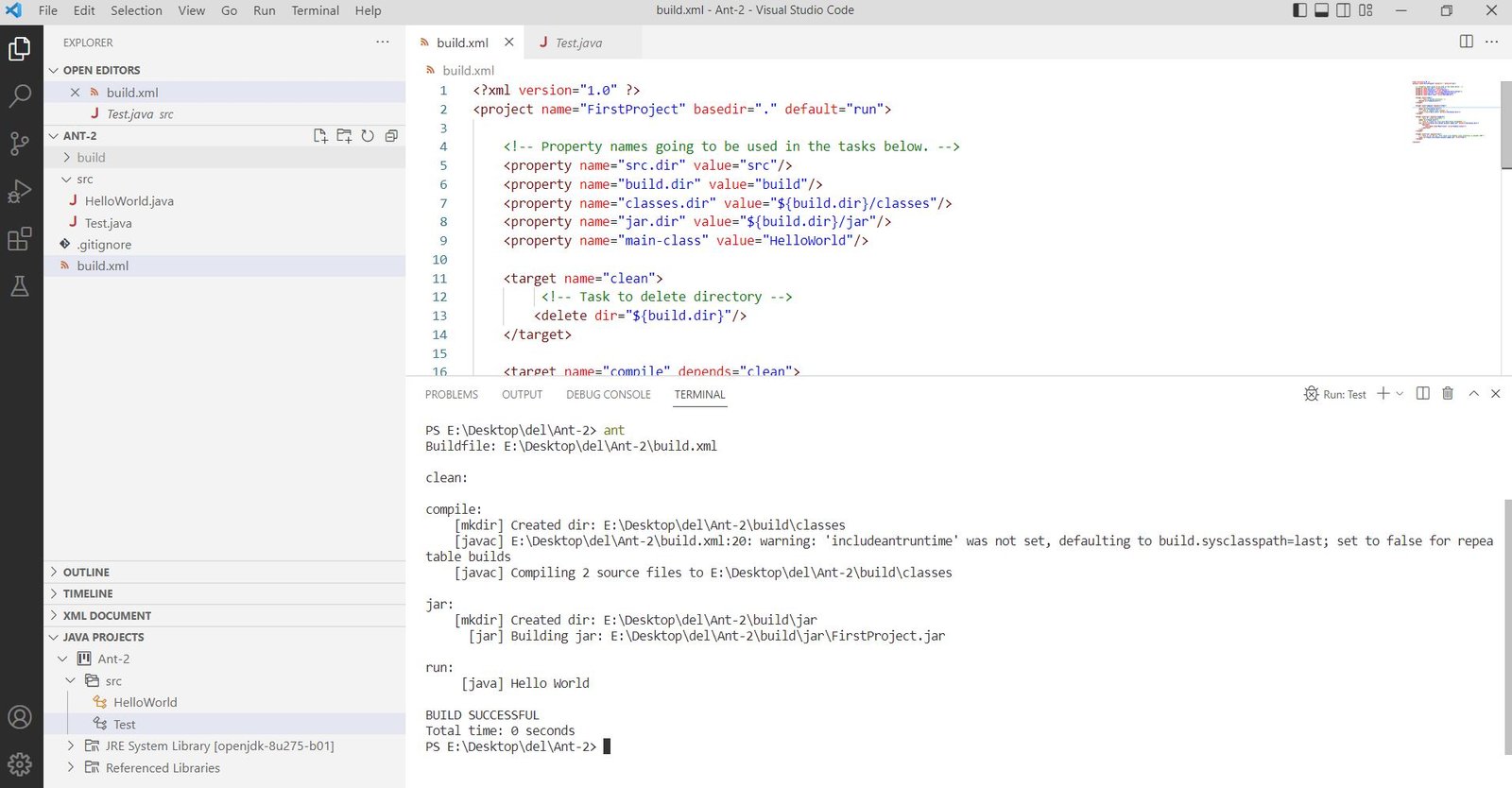
Source code:
https://github.com/it-code-lab/Ant-Sample-Script
Leave a Comment