Show List
Sample Project with JUnit Tests
Create a new maven project in IDE. Add junit-jupiter-api and junit-jupiter-engine dependencies.
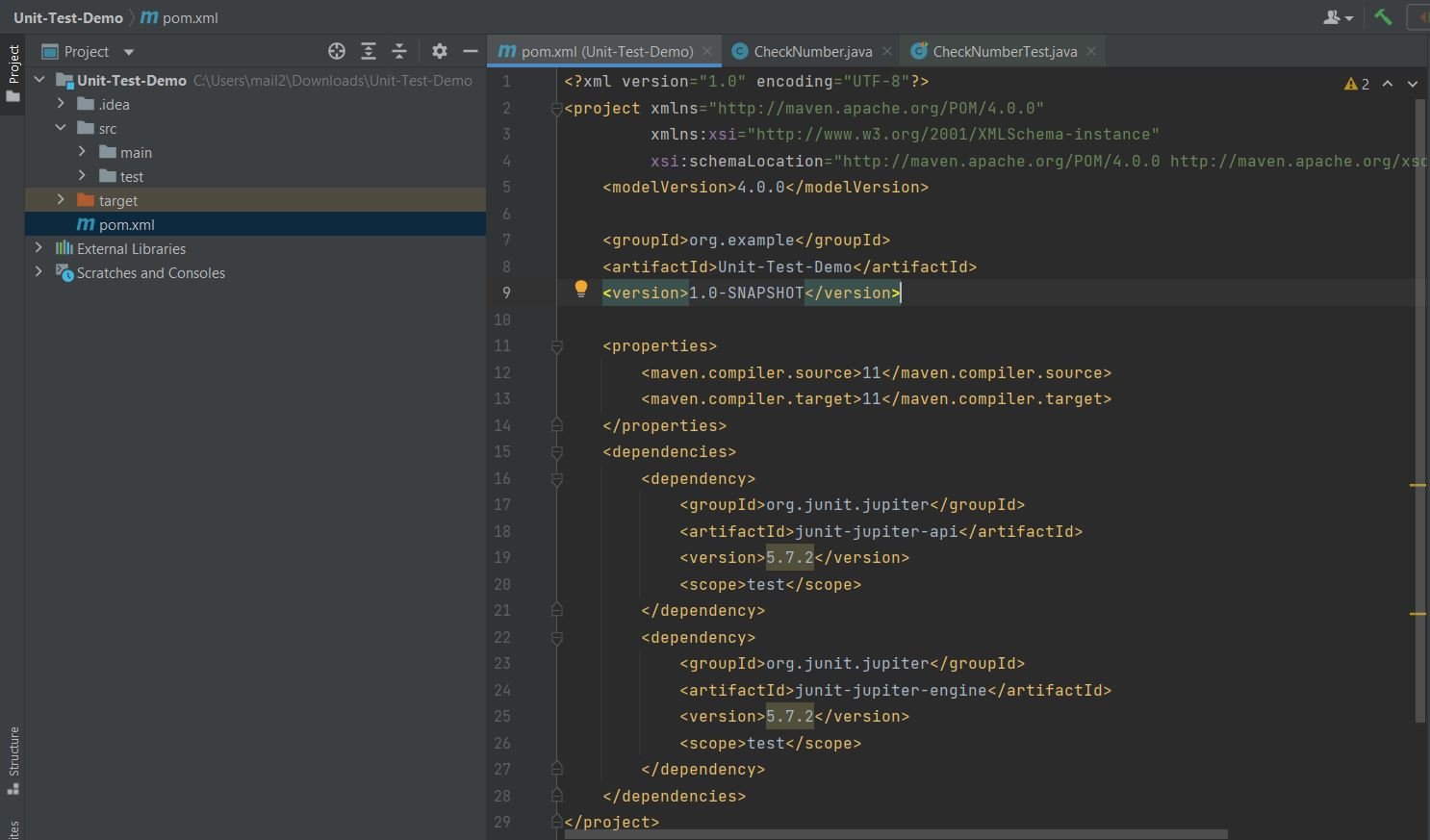
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>Unit-Test-Demo</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.7.2</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
In the src/main folder create class CheckNumber
CheckNumber.java
public class CheckNumber {
public boolean isEven(int i) {
if (i % 2 == 0) {
return true;
} else {
return false;
}
}
}
In the src/test folder create class CheckNumberTest
CheckNumberTest.java
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CheckNumberTest {
CheckNumber cn;
@BeforeEach
void setUp(){
cn = new CheckNumber();
}
@Test
void testEven(){
assertEquals(true, cn.isEven(2) );
}
@Test
void testOdd(){
assertEquals(false, cn.isEven(3) );
}
}
Common Annotations:
- @Test makes the method to be run as a test case.
- @Before method runs once before all the tests.
- @BeforeClass method is run before each test
- @After method is run once after all the tests
- @AfterClass method is run after all the tests
- @Ignore is used to ignore the test while execution
Tests can be executed individually or all together.
Run "All Tests" with coverage option shows the code coverage as well.
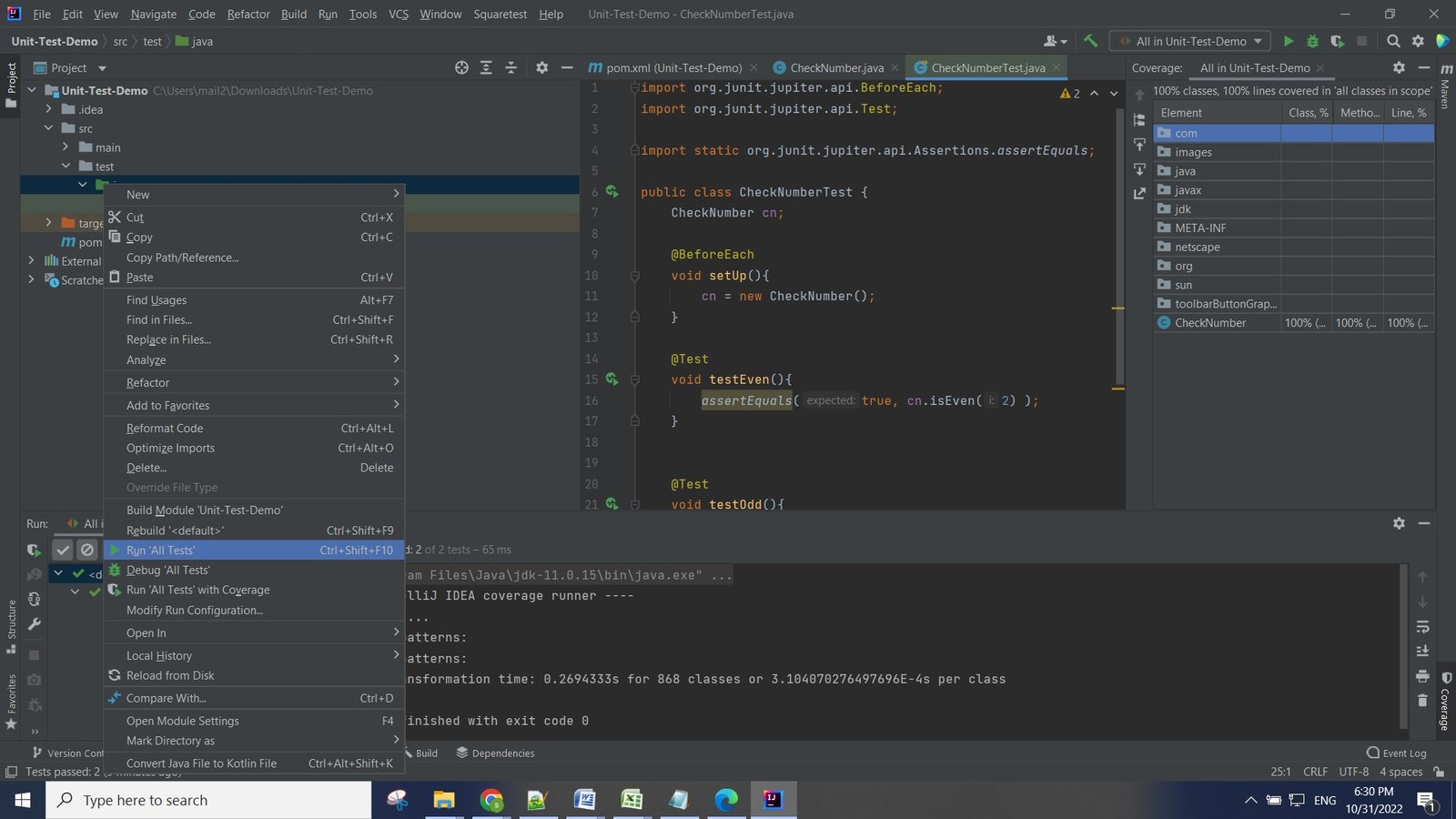
Source code:
https://github.com/it-code-lab/Unit-Test-Demo
Leave a Comment