Show List
Hibernate Example Using Annotations
Hibernate is an open-source Object Relational Mapping (ORM) framework used for mapping Java objects to relational database tables and vice versa. Using annotations in Hibernate refers to the process of defining the mapping between Java classes and database tables using annotations in the source code.
Annotations in Hibernate provide a more concise and readable way of specifying the mapping information compared to using XML configuration files. The annotations are added directly to the Java class, making the configuration easier to maintain and less prone to errors.
Here is an example of a Java class using annotations in Hibernate:
Below is the structure of the project we are going to create for this example
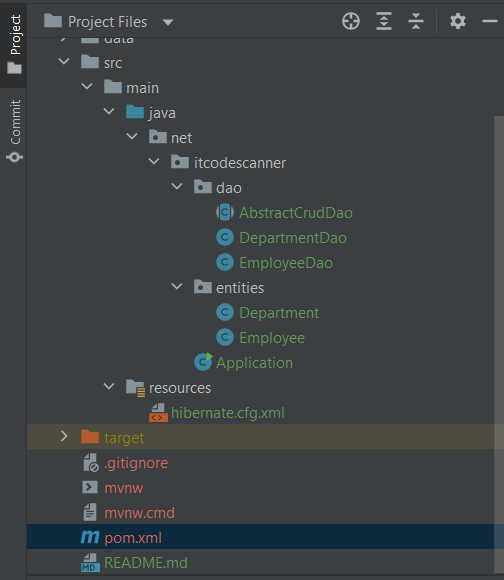
1. Create a maven project and add below hibernate-core and the selected database dependency. We are going to use h2 database for this example. pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>net.dovale.okta.spring-hibernate</groupId>
<artifactId>raw-hibernate</artifactId>
<version>1.0-SNAPSHOT</version>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>8</source>
<target>8</target>
</configuration>
</plugin>
</plugins>
</build>
<properties>
<hibernate-core-version>5.3.6.Final</hibernate-core-version>
</properties>
<dependencies>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>${hibernate-core-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.h2database/h2 -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.197</version>
</dependency>
</dependencies>
</project>
2. Create Entity classes Employee.java and Department.java in entities package
package net.itcodescanner.entities;
import javax.persistence.*;
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private int id;
private String name;
private String address;
@ManyToOne
@JoinColumn(foreignKey = @ForeignKey(name = "fk_employee_department"))
private Department department;
public Employee() {
}
public Employee( String name, String address, Department department) {
this.name = name;
this.address = address;
this.department = department;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
}
package net.itcodescanner.entities;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
public Department() {
}
public Department(String name) {
this.name = name;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
The
@Entity
annotation marks the class as a Hibernate entity, and the @Table
annotation specifies the name of the database table to which it maps. The @Id
annotation specifies that the id
property is the primary key of the table, and the @GeneratedValue
annotation specifies that the primary key value is generated automatically by the database. 3. Create Abstract Dao class AbstractCrudDao
package net.itcodescanner.dao;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import javax.persistence.criteria.CriteriaQuery;
import java.util.List;
public abstract class AbstractCrudDao<T> {
private final SessionFactory sessionFactory;
private final Class<T> entityClass;
private final String entityName;
protected AbstractCrudDao(SessionFactory sessionFactory, Class<T> entityClass, String entityName) {
this.sessionFactory = sessionFactory;
this.entityClass = entityClass;
this.entityName = entityName;
}
public T save(T entity){
sessionFactory.getCurrentSession().save(entity);
return entity;
}
public void delete(T entity){
sessionFactory.getCurrentSession().delete(entity);
}
public T find(long id){
return sessionFactory.getCurrentSession().find(entityClass, id);
}
public List<T> list(){
Session session = sessionFactory.getCurrentSession();
CriteriaQuery<T> query = session.getCriteriaBuilder().createQuery(entityClass);
query.select(query.from(entityClass));
return session.createQuery(query).getResultList();
}
}
4.Create Dao classes EmployeeDao and DepartmentDao extending AbstractCrudDao
package net.itcodescanner.dao;
import net.itcodescanner.entities.Employee;
import org.hibernate.SessionFactory;
public class EmployeeDao extends AbstractCrudDao<Employee> {
public EmployeeDao(SessionFactory sessionFactory) {
super(sessionFactory, Employee.class, "Employee");
}
}
package net.itcodescanner.dao;
import org.hibernate.SessionFactory;
public class DepartmentDao extends AbstractCrudDao<net.itcodescanner.entities.Department> {
public DepartmentDao(SessionFactory sessionFactory) {
super(sessionFactory, net.itcodescanner.entities.Department.class, "Department");
}
}
5. Create hibernate.cfg.xml providing Database connection properties, hibernate properties and mapping classes:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="connection.driver_class">org.h2.Driver</property>
<property name="connection.url">jdbc:h2:./data/db</property>
<property name="connection.username">sa</property>
<property name="connection.password"/>
<property name="dialect">org.hibernate.dialect.H2Dialect</property>
<property name="hbm2ddl.auto">create</property>
<property name="hibernate.connection.pool_size">1</property>
<property name="hibernate.current_session_context_class">thread</property>
<property name="hibernate.show_sql">true</property>
<mapping class="net.itcodescanner.entities.Employee"/>
<mapping class="net.itcodescanner.entities.Department"/>
</session-factory>
</hibernate-configuration>
6. Add the code to get the Hibernate session and call the DB methods:
package net.itcodescanner;
import net.itcodescanner.dao.*;
import net.itcodescanner.entities.*;
import org.hibernate.*;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistry;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
public class Application {
public static void main(String[] args){
StandardServiceRegistry registry = new StandardServiceRegistryBuilder().configure().build();
try (SessionFactory sessionFactory = new MetadataSources(registry).buildMetadata().buildSessionFactory()) {
EmployeeDao employeeDAO = new EmployeeDao(sessionFactory);
DepartmentDao departmentDAO = new DepartmentDao(sessionFactory);
try (Session session = sessionFactory.getCurrentSession()) {
Transaction tx = session.beginTransaction();
// create departments
Department pj = departmentDAO.save(new Department("Department-21"));
Department px = departmentDAO.save(new Department("Department-22"));
employeeDAO.save(new Employee("EmpName-1", "Address-32", pj));
employeeDAO.save(new Employee("EmpName-2", "Address-54", px));
tx.commit();
}
try (Session session = sessionFactory.getCurrentSession()) {
session.beginTransaction();
System.out.println("Employees");
employeeDAO.list().forEach(employee -> System.out.println(employee.getId() +", " + employee.getName() + ", " + employee.getAddress() + ", " + employee.getDepartment().getName() ));
System.out.println("Departments");
departmentDAO.list().forEach(department -> System.out.println(department.getName() + ", " + department.getId()));
}
}
}
}
Output:
Hibernate: drop table Department if exists Hibernate: drop table Employee if exists Hibernate: drop sequence if exists hibernate_sequence Aug 28, 2022 7:42:15 PM org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@68ed96ca] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Hibernate: create sequence hibernate_sequence start with 1 increment by 1 Hibernate: create table Department (id bigint not null, name varchar(255), primary key (id)) Hibernate: create table Employee (id integer not null, address varchar(255), name varchar(255), department_id bigint, primary key (id)) Hibernate: alter table Employee add constraint fk_employee_department foreign key (department_id) references Department Aug 28, 2022 7:42:15 PM org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@51b01960] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Aug 28, 2022 7:42:15 PM org.hibernate.tool.schema.internal.SchemaCreatorImpl applyImportSources INFO: HHH000476: Executing import script 'org.hibernate.tool.schema.internal.exec.ScriptSourceInputNonExistentImpl@4acf72b6' Hibernate: call next value for hibernate_sequence Hibernate: call next value for hibernate_sequence Hibernate: call next value for hibernate_sequence Hibernate: call next value for hibernate_sequence Hibernate: insert into Department (name, id) values (?, ?) Hibernate: insert into Department (name, id) values (?, ?) Hibernate: insert into Employee (address, department_id, name, id) values (?, ?, ?, ?) Hibernate: insert into Employee (address, department_id, name, id) values (?, ?, ?, ?) Employees Aug 28, 2022 7:42:16 PM org.hibernate.hql.internal.QueryTranslatorFactoryInitiator initiateService INFO: HHH000397: Using ASTQueryTranslatorFactory Hibernate: select employee0_.id as id1_1_, employee0_.address as address2_1_, employee0_.department_id as departme4_1_, employee0_.name as name3_1_ from Employee employee0_ Hibernate: select department0_.id as id1_0_0_, department0_.name as name2_0_0_ from Department department0_ where department0_.id=? Hibernate: select department0_.id as id1_0_0_, department0_.name as name2_0_0_ from Department department0_ where department0_.id=? 3, EmpName-1, Address-32, Department-21 4, EmpName-2, Address-54, Department-22 Departments Hibernate: select department0_.id as id1_0_, department0_.name as name2_0_ from Department department0_ Department-21, 1 Department-22, 2 Aug 28, 2022 7:42:16 PM org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl stop INFO: HHH10001008: Cleaning up connection pool [jdbc:h2:./data/db] Process finished with exit code 0
Source code:
https://github.com/it-code-lab/hibernateusingannotation
Leave a Comment