Hibernate Example Using Spring Boot
Hibernate is an Object Relational Mapping (ORM) framework for mapping Java objects to relational database tables and vice versa. Spring Boot is a framework for building production-ready applications with minimal configuration and setup. When used together, Hibernate and Spring Boot provide a powerful and flexible platform for developing database-backed applications.
Spring Boot provides support for integrating Hibernate into a Spring Boot application through the Spring Data JPA (Java Persistence API) module. This module provides a simple and straightforward way of accessing and managing data in a relational database using Hibernate. It eliminates the need for manual configuration of Hibernate and automatically creates the required beans and schema for accessing data from a database.
Here is an example of how to use Hibernate in a Spring Boot application:
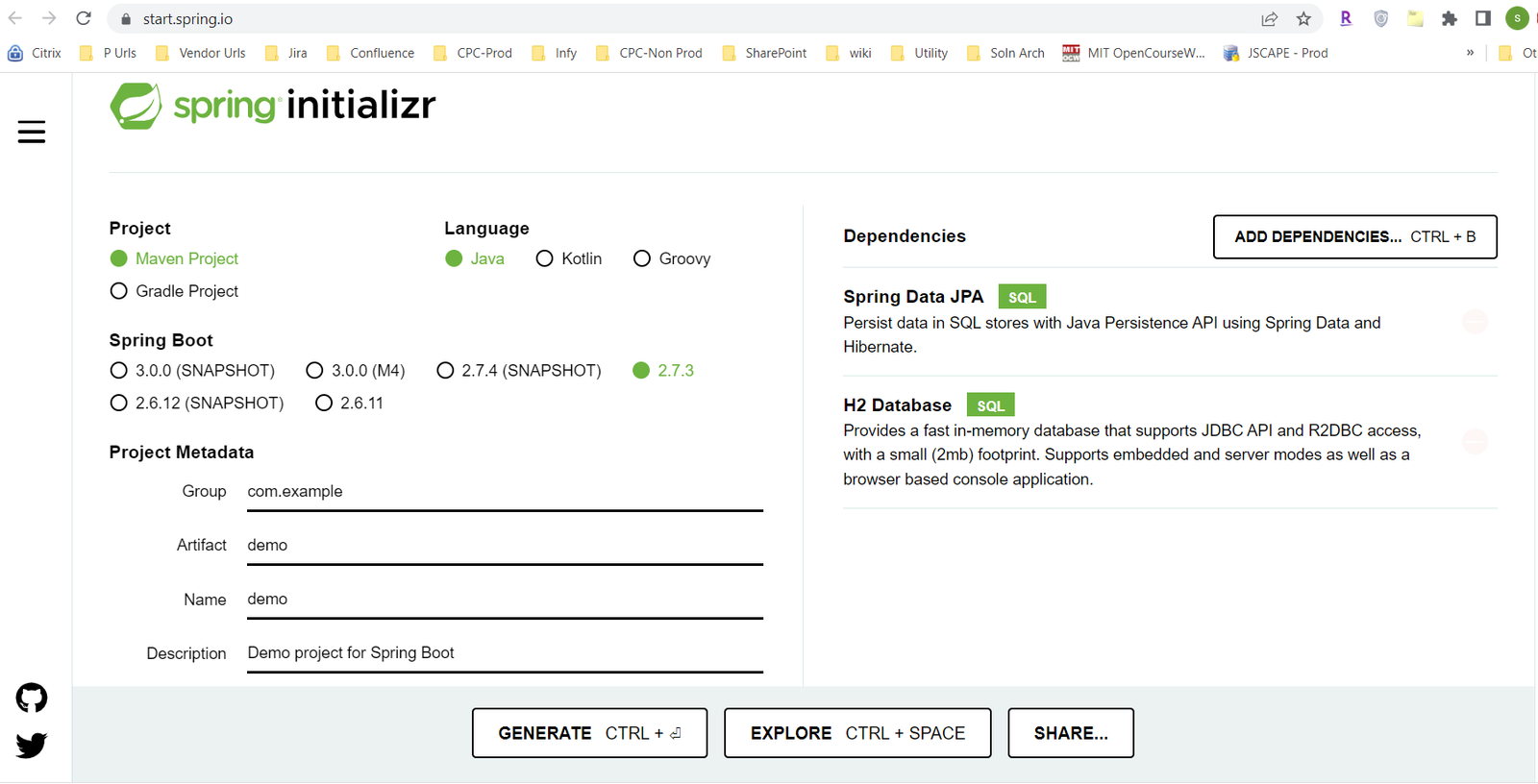
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.3</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
package net.itcodescanner.entities;
import javax.persistence.*;
@Entity
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private int id;
private String name;
private String address;
@ManyToOne
@JoinColumn(foreignKey = @ForeignKey(name = "fk_employee_department"))
private Department department;
public Employee() {
}
public Employee( String name, String address, Department department) {
this.name = name;
this.address = address;
this.department = department;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
}
package net.itcodescanner.entities;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
public Department() {
}
public Department(String name) {
this.name = name;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
package com.example.demo.dao;
import com.example.demo.entities.Employee;
import org.springframework.data.repository.CrudRepository;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Repository;
@Repository
public interface EmployeeDao extends CrudRepository<Employee, Long> {}
package com.example.demo.dao;
import com.example.demo.entities.Department;
import org.springframework.data.repository.CrudRepository;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Repository;
@Repository
public interface DepartmentDao extends CrudRepository<Department, Long> {}
package com.example.demo.service;
import com.example.demo.dao.DepartmentDao;
import com.example.demo.dao.EmployeeDao;
import com.example.demo.entities.Department;
import com.example.demo.entities.Employee;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class DataProcessingService {
@Autowired
private EmployeeDao employeeDao;
@Autowired
private DepartmentDao departmentDao;
public void insertData(){
Department d1 = new Department("Department-21");
Department d2 = new Department("Department-22");
departmentDao.save(d1);
departmentDao.save(d2);
Employee e1 = new Employee("EmpName-1", "Address-32", d1);
Employee e2 = new Employee("EmpName-2", "Address-54", d2);
employeeDao.save(e1);
employeeDao.save(e2);
}
public void printData(){
System.out.println("Employees");
employeeDao.findAll().forEach(employee -> System.out.println(employee.getId() +", " + employee.getName() + ", " + employee.getAddress() + ", " + employee.getDepartment().getName() ));
System.out.println("Departments");
departmentDao.findAll().forEach(department -> System.out.println(department.getName() + ", " + department.getId()));
}
}
package com.example.demo;
import com.example.demo.service.DataProcessingService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.transaction.annotation.EnableTransactionManagement;
@EnableTransactionManagement
@SpringBootApplication
public class DemoApplication implements CommandLineRunner {
@Autowired
private DataProcessingService s;
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
private void doDB(){
s.insertData();
s.printData();
}
@Override
public void run(String... args) throws Exception {
s.insertData();
s.printData();
}
}
Hibernate: drop table Department if exists Hibernate: drop table Employee if exists Hibernate: drop sequence if exists hibernate_sequence Aug 28, 2022 7:42:15 PM org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@68ed96ca] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Hibernate: create sequence hibernate_sequence start with 1 increment by 1 Hibernate: create table Department (id bigint not null, name varchar(255), primary key (id)) Hibernate: create table Employee (id integer not null, address varchar(255), name varchar(255), department_id bigint, primary key (id)) Hibernate: alter table Employee add constraint fk_employee_department foreign key (department_id) references Department Aug 28, 2022 7:42:15 PM org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@51b01960] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Aug 28, 2022 7:42:15 PM org.hibernate.tool.schema.internal.SchemaCreatorImpl applyImportSources INFO: HHH000476: Executing import script 'org.hibernate.tool.schema.internal.exec.ScriptSourceInputNonExistentImpl@4acf72b6' Hibernate: call next value for hibernate_sequence Hibernate: call next value for hibernate_sequence Hibernate: call next value for hibernate_sequence Hibernate: call next value for hibernate_sequence Hibernate: insert into Department (name, id) values (?, ?) Hibernate: insert into Department (name, id) values (?, ?) Hibernate: insert into Employee (address, department_id, name, id) values (?, ?, ?, ?) Hibernate: insert into Employee (address, department_id, name, id) values (?, ?, ?, ?) Employees Aug 28, 2022 7:42:16 PM org.hibernate.hql.internal.QueryTranslatorFactoryInitiator initiateService INFO: HHH000397: Using ASTQueryTranslatorFactory Hibernate: select employee0_.id as id1_1_, employee0_.address as address2_1_, employee0_.department_id as departme4_1_, employee0_.name as name3_1_ from Employee employee0_ Hibernate: select department0_.id as id1_0_0_, department0_.name as name2_0_0_ from Department department0_ where department0_.id=? Hibernate: select department0_.id as id1_0_0_, department0_.name as name2_0_0_ from Department department0_ where department0_.id=? 3, EmpName-1, Address-32, Department-21 4, EmpName-2, Address-54, Department-22 Departments Hibernate: select department0_.id as id1_0_, department0_.name as name2_0_ from Department department0_ Department-21, 1 Department-22, 2 Aug 28, 2022 7:42:16 PM org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl stop INFO: HHH10001008: Cleaning up connection pool [jdbc:h2:./data/db] Process finished with exit code 0
Leave a Comment