Show List
JDBC Example
In this example we are going to create a table in the database and then perform read operation on the table using JDBC.
Sample JDBC Java Program
Setting up the Database Table
Create the database table and insert some records. Below are some sample scripts for MySQL database table creation:
CREATE TABLE `demo`.`employees` (
`empNum` INT NOT NULL,
`empname` VARCHAR(45) NULL,
`address` VARCHAR(200) NULL,
`deptnum` INT NULL);
insert into employees values (1, 'Robert', 'address1', 12);
insert into employees values (2, 'Mary', 'address2', 12);
insert into employees values (3, 'Allen','address3', 54);
insert into employees values (4, 'Brady', 'address4', 54);
insert into employees values (5, 'Chris', 'address5', 32);
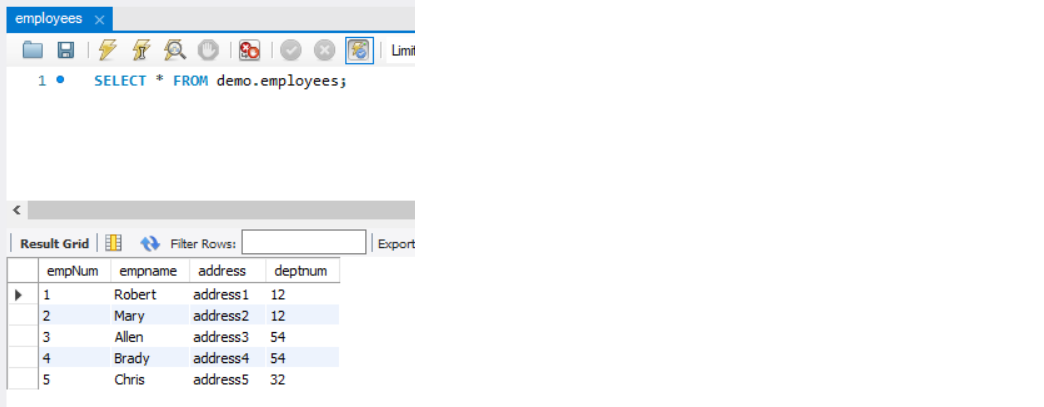
Java Program:
Download mysql-connector-java-8.0.17.jar and add to the classpath of the project.
// import sql package to use it in our program
import java.sql.*;
public class JDBCDemo {
static final String DB_URL = "jdbc:mysql://localhost:3306/demo";
static final String USER = "admin";
static final String PASS = "admin";
public static void main(String[] args) throws ClassNotFoundException, SQLException {
// SQL statement
String QUERY = "select * from employees";
//Register the driver (No longer required for new driver)
//Class.forName("com.mysql.cj.jdbc.Driver");
//Opening the connection
try(Connection conn = DriverManager.getConnection(DB_URL, USER, PASS))
{
Statement statement1 = conn.createStatement();
//Created statement and execute it
ResultSet rs1 = statement1.executeQuery(QUERY);
{
//Get the values of the record using while loop
while(rs1.next())
{
int empnum = rs1.getInt("empnum");
String empname = rs1.getString("empname");
String address = rs1.getString("address");
int deptnum = rs1.getInt("deptnum");
//store the values which are retrieved using ResultSet and print it
System.out.println(empnum + "," +empname+ "," +address+ "," +deptnum );
}
}
}
catch (SQLException e) {
//If exception occurs catch it and exit the program
e.printStackTrace();
}
}
}
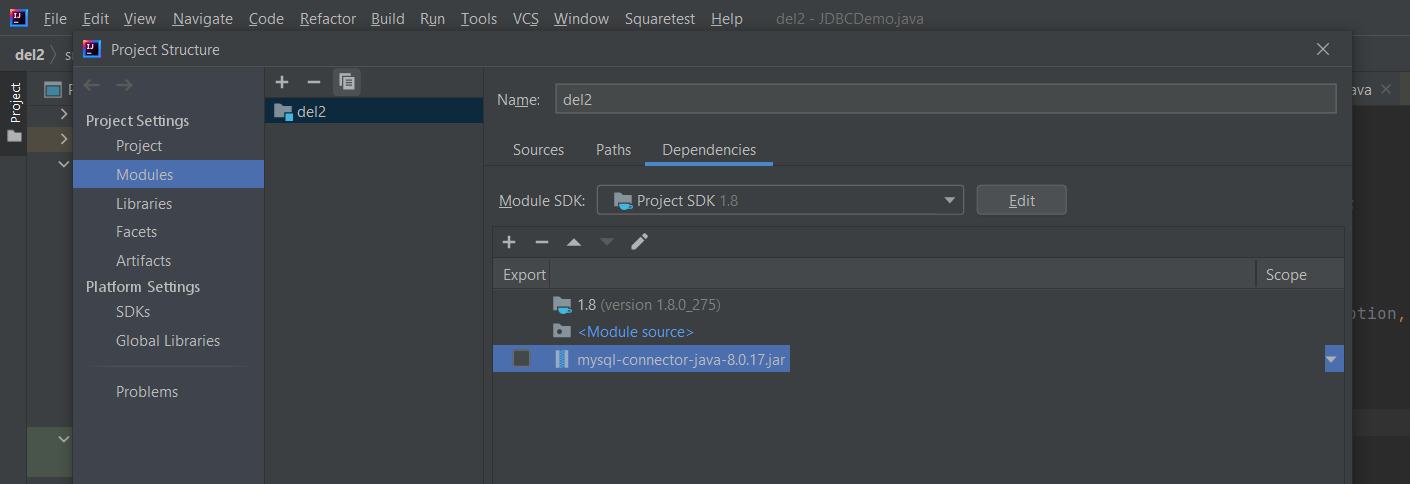
Below is the output from the program:
1,Robert,address1,12 2,Mary,address2,12 3,Allen,address3,54 4,Brady,address4,54 5,Chris,address5,32 Process finished with exit code 0
Leave a Comment