Show List
Using Gatling To Performance Test a REST API
In this demo we are going to create the test script and performance test a REST API using Gatling.
Download Gatling
Download Gatling from the official page https://gatling.io/open-source/
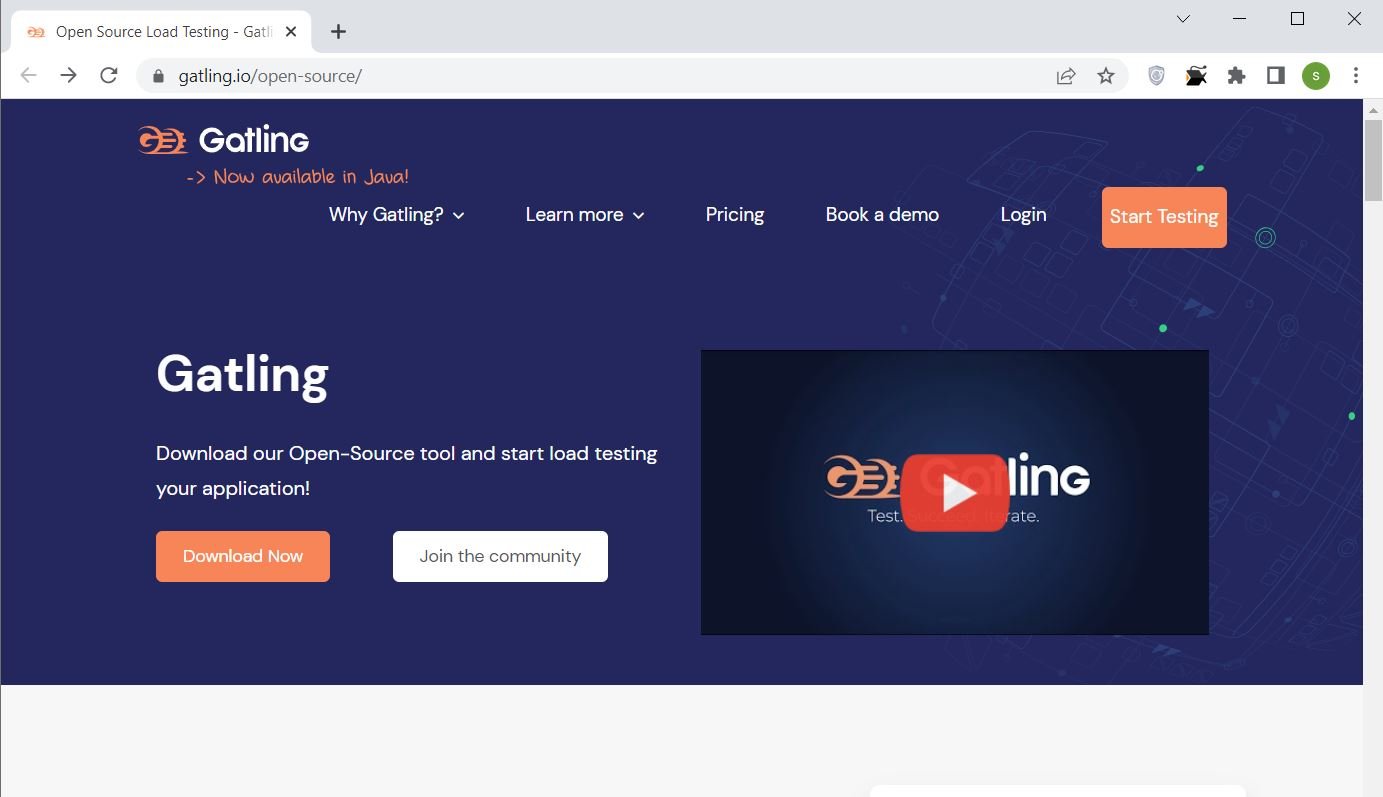
Extract the downloaded zip files into a folder:
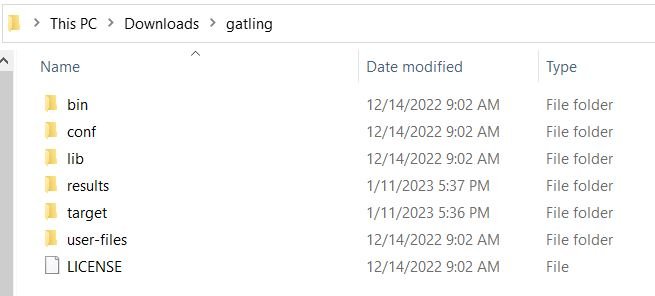
Start Script Recorder
To start the Gatling script recorder, double click the recorder.bat file in the bin directory:
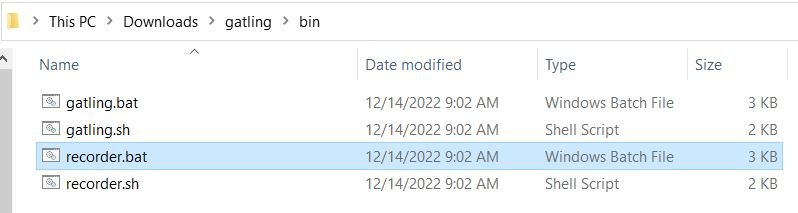
It starts the Recorder Configuration UI
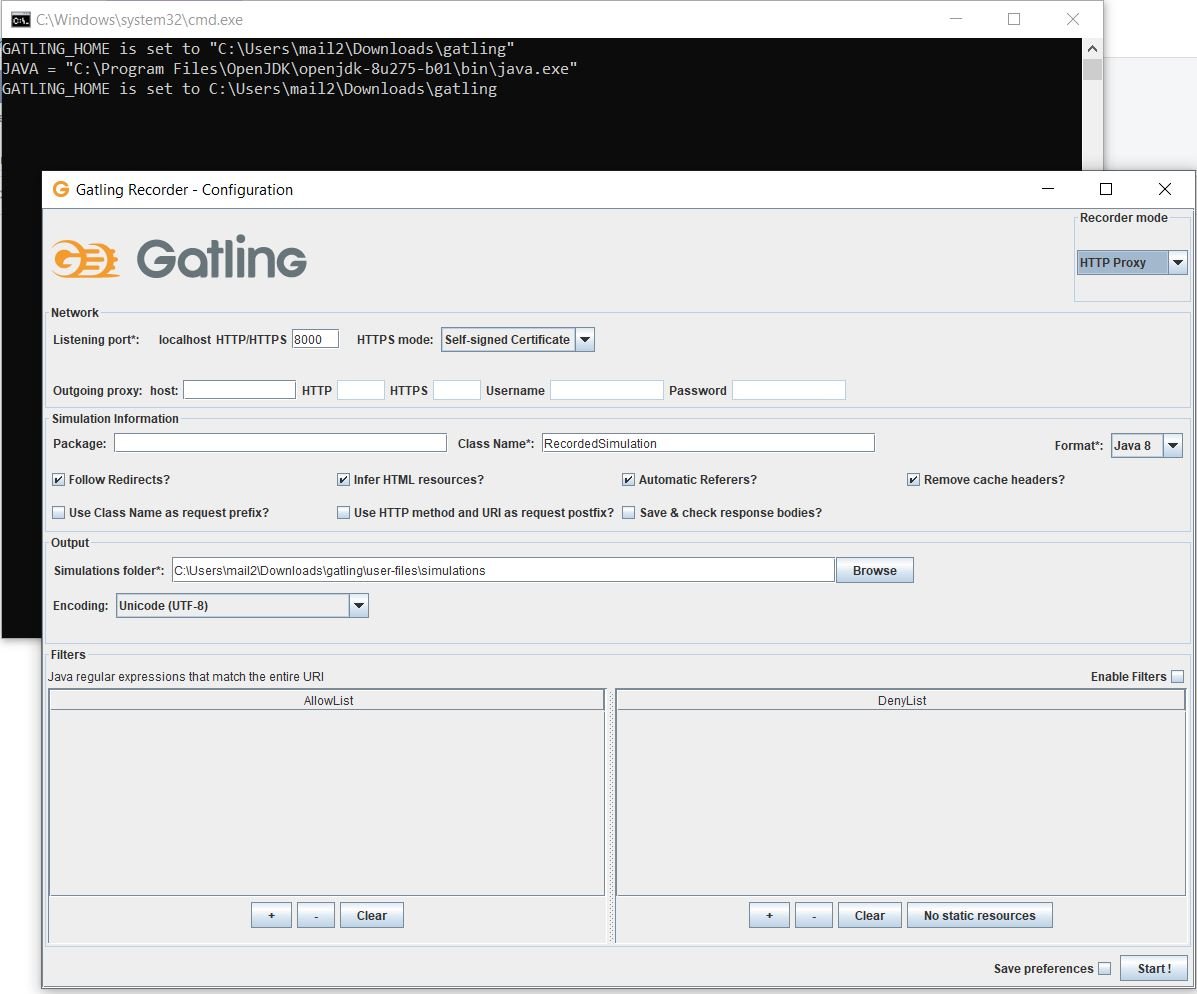
By default the recorder listens on local host port 8000 and recorder scripts would be saved under \user-files\simulations folder.
Click on the start button at the bottom right corner to turn on recording mode.
Recording the Script
We are going to use a public API for load test. To record the test script, we will use Gatling recorder as proxy on Postman while sending the request to the public API.
API End Point: http://universities.hipolabs.com/search?country=Monaco.
Here is the response from the API when the request is sent to this endpoint:
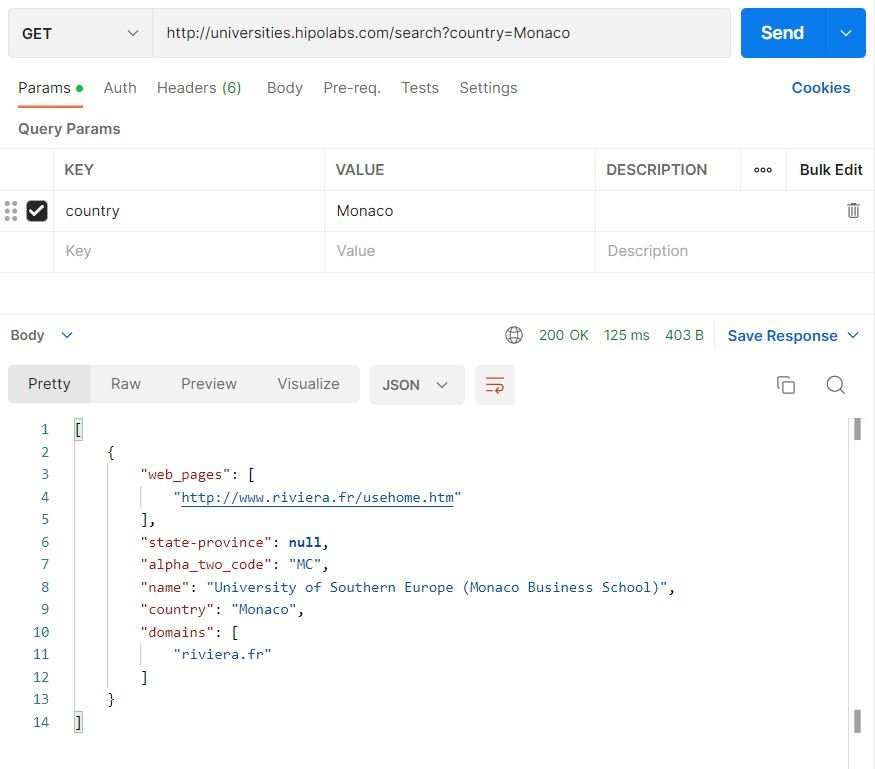
On Postman, change settings to use Gatling recorder as the proxy for the requests. To do this, click on the settings icon at the top and then go to Proxy tab and enter details as below
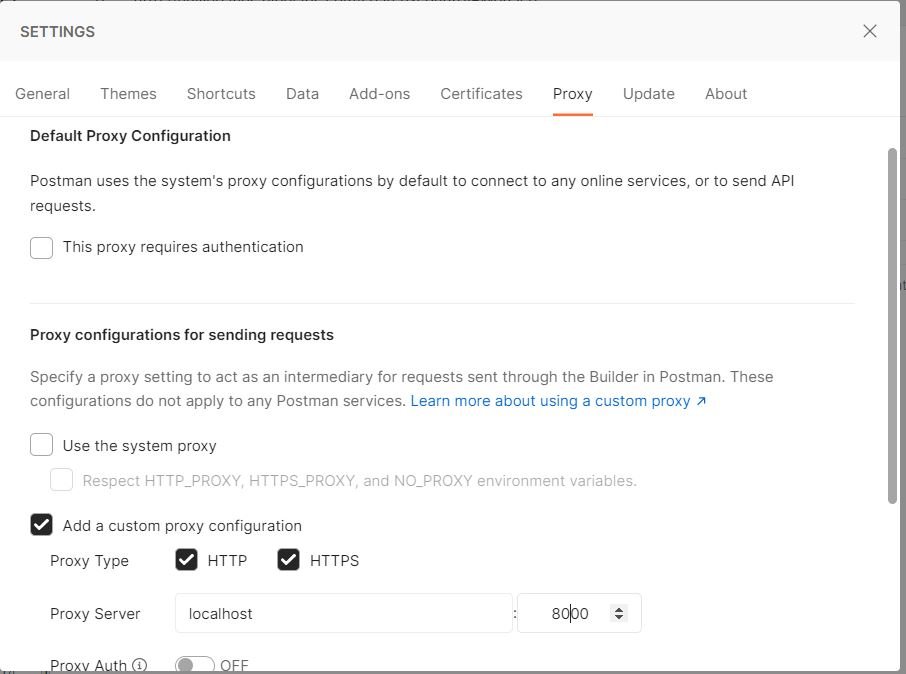
After this, when the requests are sent from the Postman, those will flow through Gatling recorder. As the recording has been turned on, the requests and response would get recorded.
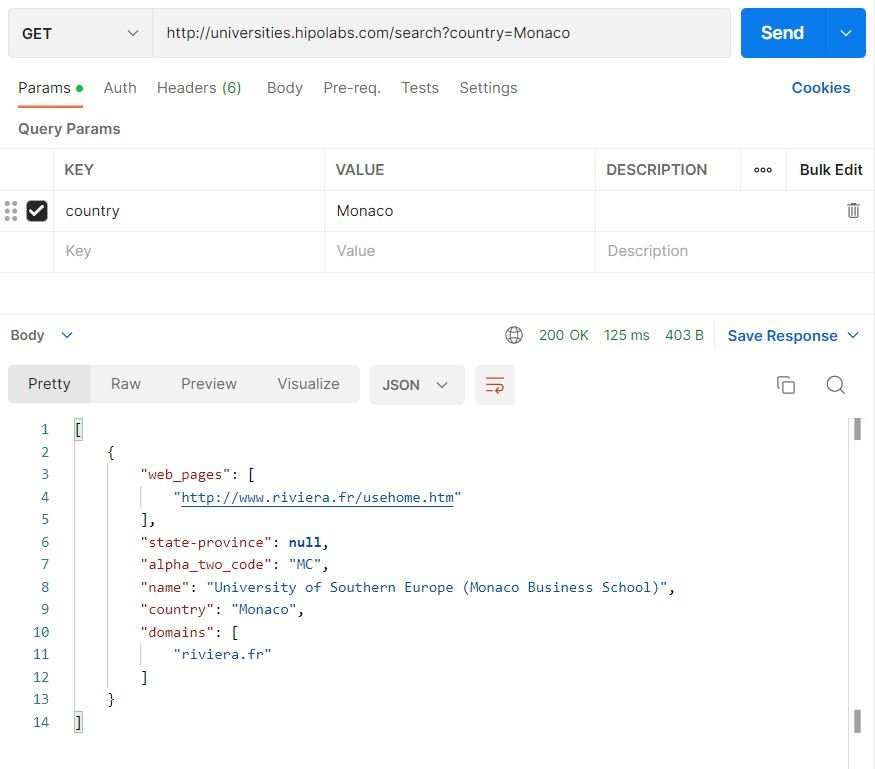
The recorder stub can be seen on the Gatling recorder UI as below. Click on the "Stop and Save" button at the top right corner to save the script.
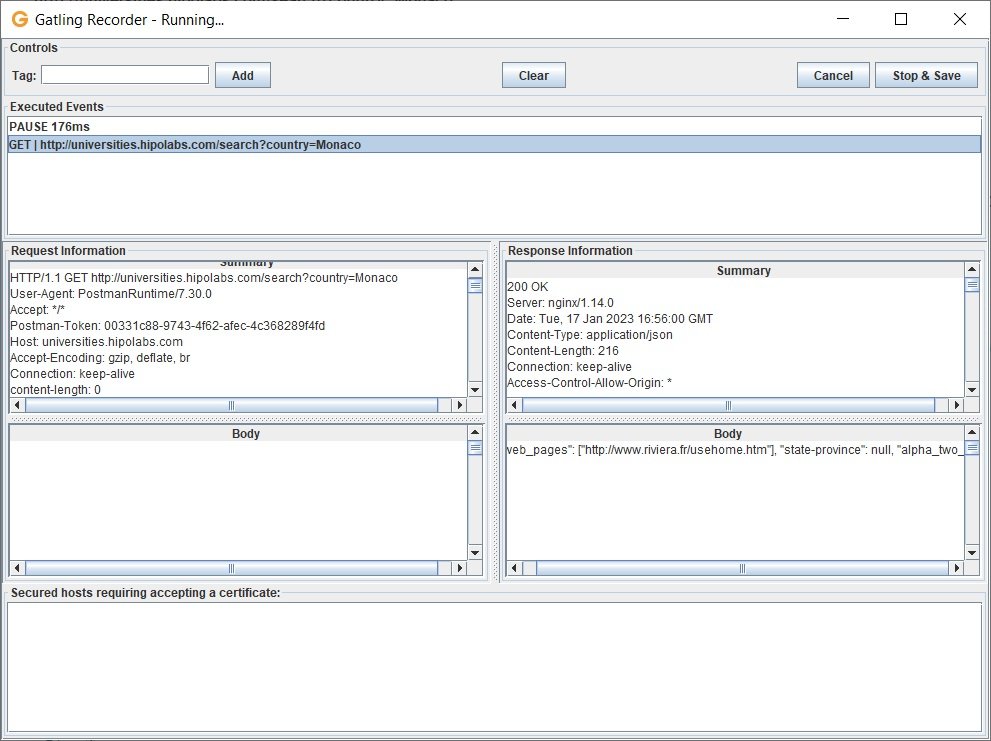
Now go to the /user-files/simulations folder to view the recorded script and to make any updates
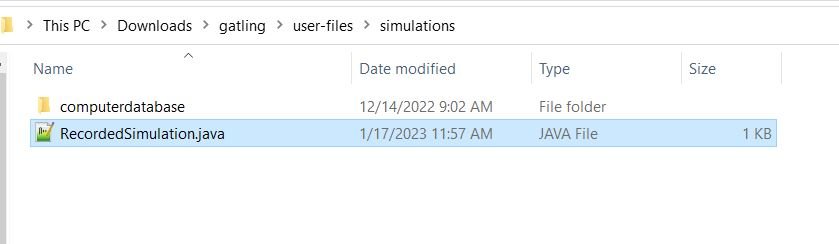
Here is the code for the script we recorded.
RecorderSimulation.java
import java.time.Duration;import java.util.*;import io.gatling.javaapi.core.*;import io.gatling.javaapi.http.*;import io.gatling.javaapi.jdbc.*;import static io.gatling.javaapi.core.CoreDsl.*;import static io.gatling.javaapi.http.HttpDsl.*;import static io.gatling.javaapi.jdbc.JdbcDsl.*;public class RecordedSimulation extends Simulation {{HttpProtocolBuilder httpProtocol = http.baseUrl("http://universities.hipolabs.com").inferHtmlResources().acceptHeader("*/*").acceptEncodingHeader("gzip, deflate").userAgentHeader("PostmanRuntime/7.30.0");Map<CharSequence, String> headers_0 = new HashMap<>();headers_0.put("Postman-Token", "00331c88-9743-4f62-afec-4c368289f4fd");ScenarioBuilder scn = scenario("RecordedSimulation").exec(http("request_0").get("/search?country=Monaco").headers(headers_0));setUp(scn.injectOpen(atOnceUsers(1))).protocols(httpProtocol);}}
HttpProtocol has the information such as the url called, information being sent in request and accepted response header etc.
ScenerioBuilder has the information about how the request is sent.
setUp defines the scenario that will be launched for the load test. atOnceUsers defines the number of requests that will be sent all together. We can have the requests spread through a period. For our demo we are going to send 5 requests per second for a period of 10 seconds. The updated script is below.
import java.time.Duration;import java.util.*;import io.gatling.javaapi.core.*;import io.gatling.javaapi.http.*;import io.gatling.javaapi.jdbc.*;import static io.gatling.javaapi.core.CoreDsl.*;import static io.gatling.javaapi.http.HttpDsl.*;import static io.gatling.javaapi.jdbc.JdbcDsl.*;public class RecordedSimulation extends Simulation {{HttpProtocolBuilder httpProtocol = http.baseUrl("http://universities.hipolabs.com").inferHtmlResources().acceptHeader("*/*").acceptEncodingHeader("gzip, deflate").userAgentHeader("PostmanRuntime/7.30.0");Map<CharSequence, String> headers_0 = new HashMap<>();headers_0.put("Postman-Token", "00331c88-9743-4f62-afec-4c368289f4fd");ScenarioBuilder scn = scenario("RecordedSimulation").exec(http("request_0").get("/search?country=Monaco").headers(headers_0));setUp(scn.injectOpen(constantUsersPerSec(5).during(Duration.ofSeconds(10)))).protocols(httpProtocol);}}
Running the Test
Double click the Gatling bat file in the bin folder. Select run locally option and press enter:
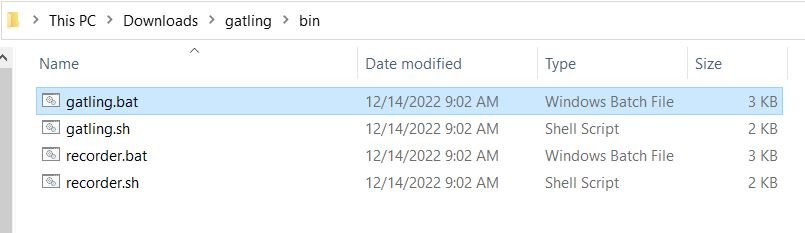
GATLING_HOME is set to "C:\Users\mail2\Downloads\gatling" JAVA = "C:\Program Files\OpenJDK\openjdk-8u275-b01\bin\java.exe" GATLING_HOME is set to C:\Users\mail2\Downloads\gatling Do you want to run the simulation locally, on Gatling Enterprise, or just package it? Type the number corresponding to your choice and press enter [0] <Quit> [1] Run the Simulation locally [2] Package and upload the Simulation to Gatling Enterprise Cloud, and run it there [3] Package the Simulation for Gatling Enterprise [4] Show help and exit 1 Choose a simulation number: [0] RecordedSimulation [1] computerdatabase.ComputerDatabaseSimulation 0 Select run description (optional) APILoadTest Simulation RecordedSimulation started... ================================================================================ 2023-01-17 12:38:19 5s elapsed ---- Requests ------------------------------------------------------------------ > Global (OK=25 KO=0 ) > request_0 (OK=25 KO=0 ) ---- RecordedSimulation -------------------------------------------------------- [##################################### ] 50% waiting: 25 / active: 0 / done: 25 ================================================================================ ================================================================================ 2023-01-17 12:38:24 9s elapsed ---- Requests ------------------------------------------------------------------ > Global (OK=50 KO=0 ) > request_0 (OK=50 KO=0 ) ---- RecordedSimulation -------------------------------------------------------- [##########################################################################]100% waiting: 0 / active: 0 / done: 50 ================================================================================ Simulation RecordedSimulation completed in 9 seconds Parsing log file(s)... Parsing log file(s) done Generating reports... ================================================================================ ---- Global Information -------------------------------------------------------- > request count 50 (OK=50 KO=0 ) > min response time 74 (OK=74 KO=- ) > max response time 109 (OK=109 KO=- ) > mean response time 83 (OK=83 KO=- ) > std deviation 6 (OK=6 KO=- ) > response time 50th percentile 82 (OK=82 KO=- ) > response time 75th percentile 85 (OK=85 KO=- ) > response time 95th percentile 93 (OK=93 KO=- ) > response time 99th percentile 101 (OK=101 KO=- ) > mean requests/sec 5 (OK=5 KO=- ) ---- Response Time Distribution ------------------------------------------------ > t < 800 ms 50 (100%) > 800 ms <= t < 1200 ms 0 ( 0%) > t ≥ 1200 ms 0 ( 0%) > failed 0 ( 0%) ================================================================================ Reports generated in 0s. Please open the following file: file:///C:/Users/mail2/Downloads/gatling/results/recordedsimulation-20230117173812791/index.html Press any key to continue . . .
Link to the test report is provided on the console.
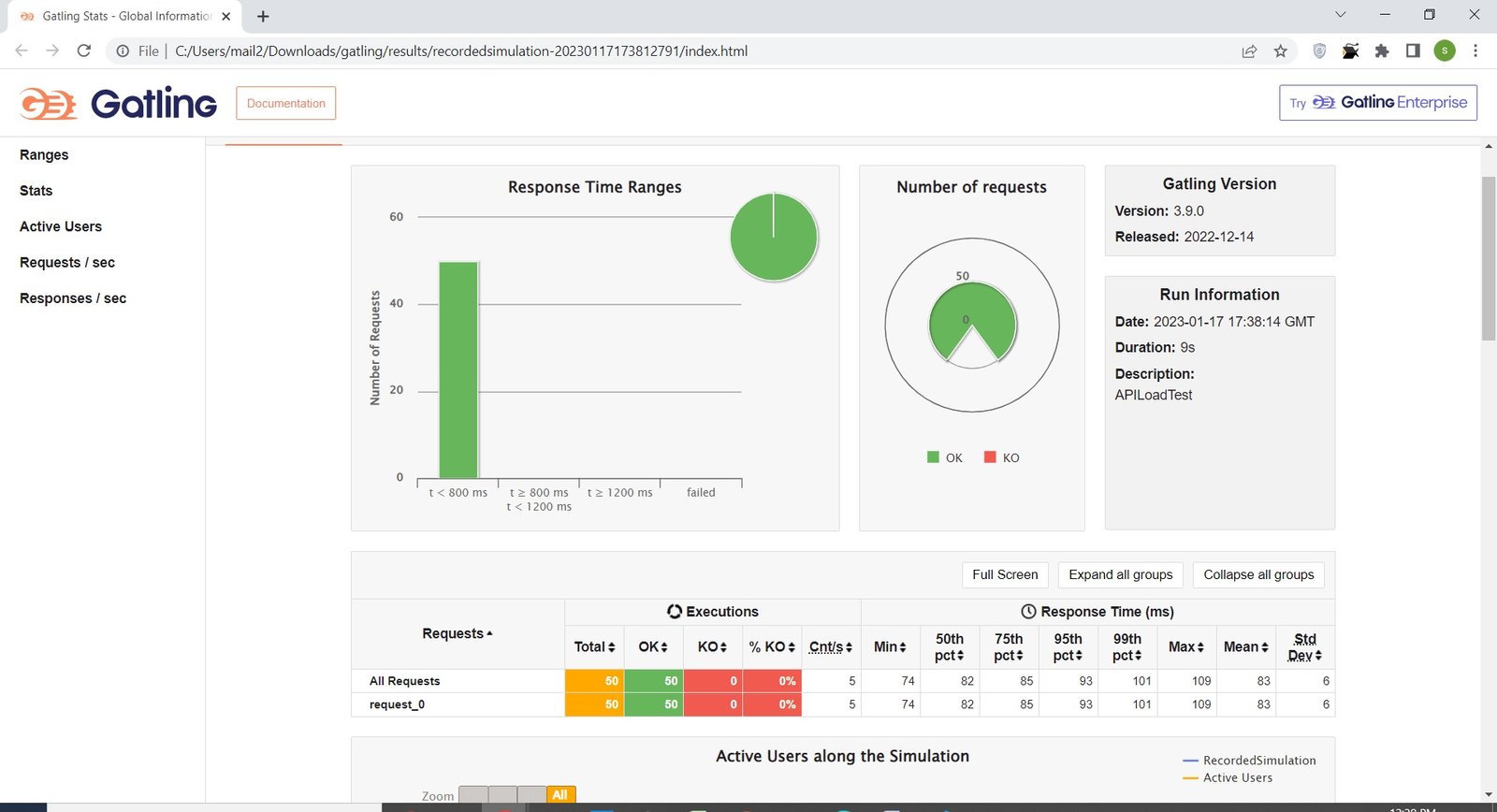
Leave a Comment